作者:じ☆ve宝贝
#### 1.下载RocketMQ-master 执行 install.bat 生成文件夹target (有问题可以去cmd去执行) #### 2.上传alibaba-rocketmq-3.2.6-alibaba-rocketmq.tar.gz 到linux服务器 #### 3.安装64位jdk #### 4.解压alibaba-rocketmq-3.2.6-alibaba-rocketmq.tar.gz ``` tar -zxvf alibaba-rocketmq-3.2.6-alibaba-rocketmq.tar.gz ``` #### 5.启动MQ ``` cd alibaba-rocketmq/bin nohup sh mqnamesrv & nohup sh mqbroker -n "10.66.66.222:9876" & more nohup.out ``` 如果显示: ``` The Name Server boot success. The broker[vdata.kt, 10.66.66.222:10911] boot success. 则NameServer,Broker启动成功 ``` #### 6.关闭MQ ``` 关闭broker sh mqshutdown broker 关闭Name Server sh mqshutdown namesrv ``` ## Producer生产者代码 ``` package ccn.studyjava.rocketmq.example; import java.util.concurrent.TimeUnit; import com.alibaba.rocketmq.client.exception.MQClientException; import com.alibaba.rocketmq.client.producer.DefaultMQProducer; import com.alibaba.rocketmq.client.producer.SendResult; import com.alibaba.rocketmq.common.message.Message; public class Producer { public static void main(String[] args) throws MQClientException, InterruptedException { /** * 一个应用创建一个Producer,由应用来维护此对象,可以设置为全局对象或者单例<br> * 注意:ProducerGroupName需要由应用来保证唯一<br> * ProducerGroup这个概念发送普通的消息时,作用不大,但是发送分布式事务消息时,比较关键, * 因为服务器会回查这个Group下的任意一个Producer */ final DefaultMQProducer producer = new DefaultMQProducer("ProducerGroupName"); producer.setNamesrvAddr("10.66.66.222:9876"); producer.setInstanceName("Producer"); /** * Producer对象在使用之前必须要调用start初始化,初始化一次即可<br> * 注意:切记不可以在每次发送消息时,都调用start方法 */ producer.start(); /** * 下面这段代码表明一个Producer对象可以发送多个topic,多个tag的消息。 * 注意:send方法是同步调用,只要不抛异常就标识成功。但是发送成功也可会有多种状态,<br> * 例如消息写入Master成功,但是Slave不成功,这种情况消息属于成功,但是对于个别应用如果对消息可靠性要求极高,<br> * 需要对这种情况做处理。另外,消息可能会存在发送失败的情况,失败重试由应用来处理。 */ for (int i = 0; i < 10; i++) { try { { Message msg = new Message("TopicTest1",// topic "TagA",// tag "OrderID001",// key ("Hello MetaQA").getBytes());// body SendResult sendResult = producer.send(msg); System.out.println(sendResult); } { Message msg = new Message("TopicTest2",// topic "TagB",// tag "OrderID0034",// key ("Hello MetaQB").getBytes());// body SendResult sendResult = producer.send(msg); System.out.println(sendResult); } { Message msg = new Message("TopicTest3",// topic "TagC",// tag "OrderID061",// key ("Hello MetaQC").getBytes());// body SendResult sendResult = producer.send(msg); System.out.println(sendResult); } } catch (Exception e) { e.printStackTrace(); } TimeUnit.MILLISECONDS.sleep(1000); } /** * 应用退出时,要调用shutdown来清理资源,关闭网络连接,从MetaQ服务器上注销自己 * 注意:我们建议应用在JBOSS、Tomcat等容器的退出钩子里调用shutdown方法 */ // producer.shutdown(); Runtime.getRuntime().addShutdownHook(new Thread(new Runnable() { public void run() { producer.shutdown(); } })); System.exit(0); } } ``` ## Consumer消费者代码 ``` package ccn.studyjava.rocketmq.example; import java.util.List; import com.alibaba.rocketmq.client.consumer.DefaultMQPushConsumer; import com.alibaba.rocketmq.client.consumer.listener.ConsumeConcurrentlyContext; import com.alibaba.rocketmq.client.consumer.listener.ConsumeConcurrentlyStatus; import com.alibaba.rocketmq.client.consumer.listener.MessageListenerConcurrently; import com.alibaba.rocketmq.client.exception.MQClientException; import com.alibaba.rocketmq.common.message.MessageExt; public class PushConsumer { /** * 当前例子是PushConsumer用法,使用方式给用户感觉是消息从RocketMQ服务器推到了应用客户端。<br> * 但是实际PushConsumer内部是使用长轮询Pull方式从MetaQ服务器拉消息,然后再回调用户Listener方法<br> */ public static void main(String[] args) throws InterruptedException, MQClientException { /** * 一个应用创建一个Consumer,由应用来维护此对象,可以设置为全局对象或者单例<br> * 注意:ConsumerGroupName需要由应用来保证唯一 */ DefaultMQPushConsumer consumer = new DefaultMQPushConsumer("ConsumerGroupName"); consumer.setNamesrvAddr("10.66.66.222:9876"); consumer.setInstanceName("Consumber"); /** * 订阅指定topic下tags分别等于TagA或TagC或TagD */ consumer.subscribe("TopicTest1", "TagA || TagC || TagD"); /** * 订阅指定topic下所有消息<br> * 注意:一个consumer对象可以订阅多个topic */ consumer.subscribe("TopicTest2", "*"); consumer.registerMessageListener(new MessageListenerConcurrently() { public ConsumeConcurrentlyStatus consumeMessage(List<MessageExt> msgs, ConsumeConcurrentlyContext context) { System.out.println(Thread.currentThread().getName() + " Receive New Messages: " + msgs.size()); MessageExt msg = msgs.get(0); if (msg.getTopic().equals("TopicTest1")) { // 执行TopicTest1的消费逻辑 if (msg.getTags() != null && msg.getTags().equals("TagA")) { // 执行TagA的消费 System.out.println(new String(msg.getBody())); } else if (msg.getTags() != null && msg.getTags().equals("TagC")) { // 执行TagC的消费 System.out.println(new String(msg.getBody())); } else if (msg.getTags() != null && msg.getTags().equals("TagD")) { // 执行TagD的消费 System.out.println(new String(msg.getBody())); } } else if (msg.getTopic().equals("TopicTest2")) { System.out.println(new String(msg.getBody())); } return ConsumeConcurrentlyStatus.CONSUME_SUCCESS; } }); /** * Consumer对象在使用之前必须要调用start初始化,初始化一次即可<br> */ consumer.start(); System.out.println("Consumer Started."); } } ``` ## Maven jar包 ``` <!-- rocketMQ Start --> <dependency> <groupId>com.alibaba.rocketmq</groupId> <artifactId>rocketmq-all</artifactId> <version>3.0.4-open</version> <type>pom</type> </dependency> <dependency> <groupId>com.alibaba.rocketmq</groupId> <artifactId>rocketmq-client</artifactId> <version>3.0.4-open</version> </dependency> <dependency> <groupId>com.alibaba.rocketmq</groupId> <artifactId>rocketmq-common</artifactId> <version>3.0.4-open</version> </dependency> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-classic</artifactId> <version>1.1.1</version> </dependency> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-core</artifactId> <version>1.1.1</version> </dependency> <!-- rocketMQ End --> ```
作者:微信小助手
<p style="max-width: 100%;min-height: 1em;text-align: center;box-sizing: border-box !important;overflow-wrap: break-word !important;" data-mpa-powered-by="yiban.io"><span style="max-width: 100%;color: rgb(136, 136, 136);font-family: Georgia, "Times New Roman", Times, "Songti SC", serif;font-size: 14px;letter-spacing: 0.544px;box-sizing: border-box !important;overflow-wrap: break-word !important;">点击蓝色“</span><span style="max-width: 100%;font-family: Georgia, "Times New Roman", Times, "Songti SC", serif;font-size: 14px;letter-spacing: 0.544px;color: rgb(0, 128, 255);box-sizing: border-box !important;overflow-wrap: break-word !important;">程序猿DD</span><span style="max-width: 100%;color: rgb(136, 136, 136);font-family: Georgia, "Times New Roman", Times, "Songti SC", serif;font-size: 14px;letter-spacing: 0.544px;box-sizing: border-box !important;overflow-wrap: break-word !important;">”关注我</span></p> <p style="max-width: 100%;min-height: 1em;white-space: normal;text-align: center;box-sizing: border-box !important;overflow-wrap: break-word !important;"><span style="max-width: 100%;color: rgb(136, 136, 136);font-family: Georgia, "Times New Roman", Times, "Songti SC", serif;font-size: 14px;letter-spacing: 0.544px;box-sizing: border-box !important;overflow-wrap: break-word !important;">回复“</span><span style="max-width: 100%;font-family: Georgia, "Times New Roman", Times, "Songti SC", serif;font-size: 14px;letter-spacing: 0.544px;color: rgb(0, 128, 255);box-sizing: border-box !important;overflow-wrap: break-word !important;">资源</span><span style="max-width: 100%;color: rgb(136, 136, 136);font-family: Georgia, "Times New Roman", Times, "Songti SC", serif;font-size: 14px;letter-spacing: 0.544px;box-sizing: border-box !important;overflow-wrap: break-word !important;">”获取独家整理的学习资料!</span></p> <p style="max-width: 100%;min-height: 1em;white-space: normal;text-align: center;box-sizing: border-box !important;overflow-wrap: break-word !important;"><br></p> <p style="max-width: 100%;min-height: 1em;white-space: normal;text-align: center;box-sizing: border-box !important;overflow-wrap: break-word !important;"><img class="" data-ratio="0.5625" src="/upload/ec765a9f363a6f1c971b67d24289b86c.jpg" data-type="jpeg" data-w="640"><br style="max-width: 100%;font-family: -apple-system-font, system-ui, "Helvetica Neue", "PingFang SC", "Hiragino Sans GB", "Microsoft YaHei UI", "Microsoft YaHei", Arial, sans-serif;letter-spacing: 0.544px;background-color: rgb(255, 255, 255);box-sizing: border-box !important;overflow-wrap: break-word !important;"></p> <p style="max-width: 100%;min-height: 1em;white-space: normal;text-align: center;box-sizing: border-box !important;overflow-wrap: break-word !important;"><br></p> <p style="box-sizing: border-box;margin-bottom: 15px;font-size: 16px;white-space: pre-line;line-height: 30px;color: rgb(74, 74, 74);font-family: Avenir, -apple-system-font, 微软雅黑, sans-serif;text-align: start;">很早以前,在刚开始搞Spring Cloud基础教程的时候,写过这样一篇文章:《微服务架构的基础框架选择:Spring Cloud还是Dubbo?》,可能不少读者也都看过。之后也就一直有关于这两个框架怎么选的问题出来,其实文中我有明确的提过,Spring Cloud与Dubbo的比较本身是不公平的,主要前者是一套较为完整的架构方案,而Dubbo只是服务治理与RPC实现方案。</p> <p style="box-sizing: border-box;margin-top: 15px;margin-bottom: 15px;font-size: 16px;white-space: pre-line;line-height: 30px;color: rgb(74, 74, 74);font-family: Avenir, -apple-system-font, 微软雅黑, sans-serif;text-align: start;">由于Dubbo在国内有着非常大的用户群体,但是其周边设施与组件相对来说并不那么完善。很多开发者用户又很希望享受Spring Cloud的生态,因此也会有一些Spring Cloud与Dubbo一起使用的案例与方法出现,但是一直以来大部分Spring Cloud整合Dubbo的使用方案都比较别扭。这主要是由于Dubbod的注册中心采用了ZooKeeper,而开始时Spring Cloud体系中的注册中心并不支持ZooKeeper,所以很多方案是存在两个不同注册中心的,之后即使Spring Cloud支持了ZooKeeper,但是由于服务信息的粒度与存储也不一致。所以,长期以来,在服务治理层面上,这两者一直都一套完美的融合方案。</p> <p style="box-sizing: border-box;margin-top: 15px;margin-bottom: 15px;font-size: 16px;white-space: pre-line;line-height: 30px;color: rgb(74, 74, 74);font-family: Avenir, -apple-system-font, 微软雅黑, sans-serif;text-align: start;">直到Spring Cloud Alibaba的出现,才得以解决这样的问题。在之前的教程中,我们已经介绍过使用Spring Cloud Alibaba中的Nacos来作为服务注册中心,并且在此之下可以如传统的Spring Cloud应用一样地使用Ribbon或Feign来实现服务消费。这篇,我们就来继续说说Spring Cloud Alibaba中特别支持的RPC方案:Dubbo。</p> <h2 style="box-sizing: border-box;margin-top: 1.5rem;margin-bottom: 1rem;color: rgb(21, 153, 87);line-height: 1.35;font-size: 24px;text-align: start;white-space: normal;font-family: Menlo, Monaco, "Source Code Pro", Consolas, Inconsolata, "Ubuntu Mono", "DejaVu Sans Mono", "Courier New", "Droid Sans Mono", "Hiragino Sans GB", 微软雅黑, monospace !important;"><strong><span style="font-size: 20px;">入门案例</span></strong></h2> <p style="box-sizing: border-box;margin-top: 15px;margin-bottom: 15px;font-size: 16px;white-space: pre-line;line-height: 30px;color: rgb(74, 74, 74);font-family: Avenir, -apple-system-font, 微软雅黑, sans-serif;text-align: start;">我们先通过一个简单的例子,来直观地感受Nacos服务注册中心之下,利用Dubbo来实现服务提供方与服务消费方。这里省略Nacos的安装与使用,如果对Nacos还不了解,可以查看本系列的使用Nacos实现服务注册与发现,下面就直接进入Dubbo的使用步骤。</p> <h3 style="box-sizing: border-box;margin-top: 1.5rem;margin-bottom: 1rem;color: rgb(21, 153, 87);line-height: 1.35;font-size: 20px;text-align: start;white-space: normal;font-family: Menlo, Monaco, "Source Code Pro", Consolas, Inconsolata, "Ubuntu Mono", "DejaVu Sans Mono", "Courier New", "Droid Sans Mono", "Hiragino Sans GB", 微软雅黑, monospace !important;"><span style="font-size: 18px;">构建服务接口</span></h3> <p style="box-sizing: border-box;margin-top: 15px;margin-bottom: 15px;font-size: 16px;white-space: pre-line;line-height: 30px;color: rgb(74, 74, 74);font-family: Avenir, -apple-system-font, 微软雅黑, sans-serif;text-align: start;">创建一个简单的Java项目,并在下面定义一个抽象接口,比如:</p> <pre class="prettyprint linenums prettyprinted" style="box-sizing: border-box;padding-top: 8px;padding-bottom: 6px;background: rgb(241, 239, 238);border-radius: 0px;overflow-y: auto;color: rgb(80, 97, 109);text-align: start;font-size: 10px;line-height: 12px;font-family: consolas, menlo, courier, monospace, "Microsoft Yahei"!important;border-width: 1px !important;border-style: solid !important;border-color: rgb(226, 226, 226) !important;"> <ol class="linenums list-paddingleft-2" style="list-style-type: none;"> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="java language-java" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="kwd" style="box-sizing: border-box;color: rgb(102, 102, 234);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">public</span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="kwd" style="box-sizing: border-box;color: rgb(102, 102, 234);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">interface</span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="typ" style="box-sizing: border-box;color: rgb(64, 126, 231);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">HelloService</span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="pun" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">{</span></code></span></span></p></li> <li><p><br></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="java language-java" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="typ" style="box-sizing: border-box;color: rgb(64, 126, 231);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">String</span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> hello</span><span class="pun" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">(</span><span class="typ" style="box-sizing: border-box;color: rgb(64, 126, 231);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">String</span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> name</span><span class="pun" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">);</span></code></span></span></p></li> <li><p><br></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="java language-java" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pun" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">}</span></code></span></span></p></li> </ol></pre> <h3 style="box-sizing: border-box;margin-top: 1.5rem;margin-bottom: 1rem;color: rgb(21, 153, 87);line-height: 1.35;font-size: 20px;text-align: start;white-space: normal;font-family: Menlo, Monaco, "Source Code Pro", Consolas, Inconsolata, "Ubuntu Mono", "DejaVu Sans Mono", "Courier New", "Droid Sans Mono", "Hiragino Sans GB", 微软雅黑, monospace !important;"><span style="font-size: 18px;">构建服务接口提供方</span></h3> <p style="box-sizing: border-box;margin-top: 15px;margin-bottom: 15px;font-size: 16px;white-space: pre-line;line-height: 30px;color: rgb(74, 74, 74);font-family: Avenir, -apple-system-font, 微软雅黑, sans-serif;text-align: start;"><strong style="box-sizing: border-box;color: rgb(0, 0, 0);">第一步</strong>:创建一个Spring Boot项目,在 <code class="prettyprint code-in-text prettyprinted" style="box-sizing: border-box;background: rgb(243, 241, 241);color: rgb(88, 88, 88);line-height: 18px;font-family: consolas, menlo, courier, monospace, "Microsoft Yahei"!important;border-width: 0px !important;border-style: initial !important;border-color: initial !important;"><span class="pln" style="box-sizing: border-box;background-image: initial;background-position: initial;background-size: initial;background-repeat: initial;background-attachment: initial;background-origin: initial;background-clip: initial;display: inline-block;padding-right: 2px;padding-left: 2px;font-size: 14px;">pom</span><span class="pun" style="box-sizing: border-box;background-image: initial;background-position: initial;background-size: initial;background-repeat: initial;background-attachment: initial;background-origin: initial;background-clip: initial;display: inline-block;padding-right: 2px;padding-left: 2px;font-size: 14px;">.</span><span class="pln" style="box-sizing: border-box;background-image: initial;background-position: initial;background-size: initial;background-repeat: initial;background-attachment: initial;background-origin: initial;background-clip: initial;display: inline-block;padding-right: 2px;padding-left: 2px;font-size: 14px;">xml</span></code>中引入第一步中构建的API包以及Spring Cloud Alibaba对Nacos和Dubbo的依赖,比如:</p> <pre class="prettyprint linenums prettyprinted" style="box-sizing: border-box;padding-top: 8px;padding-bottom: 6px;background: rgb(241, 239, 238);border-radius: 0px;overflow-y: auto;color: rgb(80, 97, 109);text-align: start;font-size: 10px;line-height: 12px;font-family: consolas, menlo, courier, monospace, "Microsoft Yahei"!important;border-width: 1px !important;border-style: solid !important;border-color: rgb(226, 226, 226) !important;"> <ol class="linenums list-paddingleft-2" style="list-style-type: none;"> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><dependencies></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="com" style="box-sizing: border-box;color: rgb(156, 148, 145);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><!-- 第一步中构建的API包 --></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><dependency></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><groupId></span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">com.didispace</span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></groupId></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><artifactId></span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">alibaba-dubbo-api</span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></artifactId></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><version></span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">0.0.1-SNAPSHOT</span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></version></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></dependency></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><dependency></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><groupId></span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">org.springframework.boot</span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></groupId></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><artifactId></span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">spring-boot-starter-actuator</span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></artifactId></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></dependency></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><dependency></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><groupId></span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">org.springframework.boot</span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></groupId></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><artifactId></span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">spring-boot-starter-web</span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></artifactId></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></dependency></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><dependency></span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="com" style="box-sizing: border-box;color: rgb(156, 148, 145);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><!--<groupId>com.alibaba.cloud</groupId>--></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><groupId></span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">org.springframework.cloud</span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></groupId></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><artifactId></span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">spring-cloud-starter-dubbo</span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></artifactId></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></dependency></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><dependency></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><groupId></span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">org.springframework.cloud</span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></groupId></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"><artifactId></span><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;">spring-cloud-starter-alibaba-nacos-discovery</span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></artifactId></span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></dependency></span></code></span></span></p></li> <li><p><br></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> //...</span></code></span></span></p></li> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);display: block;line-height: 22px;font-size: 14px !important;word-break: inherit !important;"><span style="box-sizing: border-box;line-height: 22px;display: block;word-break: inherit !important;"><code class="xml language-xml" style="box-sizing: border-box;margin-left: -20px;display: flex;overflow: initial;line-height: 12px;overflow-wrap: normal;border-width: 0px;border-style: initial;border-color: initial;font-size: 10px;font-family: inherit !important;white-space: pre !important;"><span class="pln" style="box-sizing: border-box;color: rgb(27, 25, 24);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"> </span><span class="tag" style="box-sizing: border-box;color: rgb(242, 44, 64);line-height: 20px;font-size: 13px !important;white-space: inherit !important;"></dependencies></span></code></span></span></p></li> </ol></pre> <p style="box-sizing: border-box;margin-top: 15px;margin-bottom: 15px;font-size: 16px;white-space: pre-line;line-height: 30px;color: rgb(74, 74, 74);font-family: Avenir, -apple-system-font, 微软雅黑, sans-serif;text-align: start;">这里需要注意两点:</p> <ol style="" class=" list-paddingleft-2"> <li><p><span style="box-sizing: border-box;color: rgb(74, 74, 74);line-height: 22px;font-size: 14px !important;"><span style="box-sizing: border-box;line-height: 22px;">必须包含 <code class="prettyprint code-in-text prettyprinted" style="box-sizing: border-box;background: rgb(243, 241, 241);color: rgb(88, 88, 88);font-size: 16px;line-height: 18px;font-family: consolas, menlo, courier, monospace, "Microsoft Yahei"!important;border-width: 0px !important;border-style: initial !important;border-color: initial !important;"><span class="pln" style="box-sizing: border-box;background-image: initial;background-position: initial;background-size: initial;background-repeat: initial;background-attachment: initial;background-origin: initial;background-clip: initial;display: inline-block;padding-right: 2px;padding-left: 2px;line-height: 22px;font-size: 14px !important;">spring</span><span class="pun" style="box-sizing: border-box;background-image: initial;background-position: initial;background-size: initial;background-repeat: initial;background-attachment: initial;background-origin: initial;background-clip: initial;display: inline-block;padding-right: 2px;padding-left: 2px;line-height: 22px;font-size: 14px !important;">-</span><span class="pln" style="box-sizing: border-box;background-image: initial;background-position: initial;background-size: initial;background-repeat: initial;background-attachment: initial;background-origin: initial;background-clip: initial;display: inline-block;padding-right: 2px;padding-left: 2px;line-height: 22px;font-size: 14px !important;">boot</span><span class="pun" style="box-sizing: border-box;background-image: initial;background-position: initial;background-size: initial;background-repeat: initial;background-attachment: initial;background-origi
作者:じ☆ve宝贝
> 在开源中国上看到《Jib 1.0.0 GA 发布:构建 Java Docker 镜像从未如此简单》,目的是为了更方便将 Java 应用程序容器化,Google 于去年推出了 Jib 工具。Jib 是一个快速而简单的容器镜像构建工具,它负责处理将应用程序打包到容器镜像中所需的所有步骤。 ## 项目git地址: 码云: https://gitee.com/mirrors/Jib github:https://github.com/GoogleContainerTools/jib ## 构建java环境 以 Maven 和 Gradle 插件形式提供(本文仅提供maven的使用方式) 在pom.xml中增加 ``` <build> <plugins> .... <plugin> <groupId>com.google.cloud.tools</groupId> <artifactId>jib-maven-plugin</artifactId> <version>1.0.0</version> <configuration> <from> <image>registry.cn-beijing.aliyuncs.com/studyjava/test:1.0.0</image> <auth> <username>阿里云登录用户名</username> <password>阿里云容器管理页面设置的Registry登录密码</password> </auth> </from> <to> <image>registry.cn-beijing.aliyuncs.com/studyjava/study:1.0.0</image> <auth> <username>阿里云登录用户名</username> <password>阿里云容器管理页面设置的Registry登录密码</password> </auth> </to> </configuration> <executions> <execution> <phase>package</phase> <goals> <goal>build</goal> </goals> </execution> </executions> </plugin> ... </plugins> </build> ``` ## 部分参数介绍 ``` from:拉取镜像的配置,默认为gcr.io/distroless/java 改地址被墙,下面会讲解如何通过阿里云代理拉去该镜像 to:要生成的镜像的配置 image:拉取或生成的镜像名称 auth: 认证信息,分别为用户名和密码 <!-- 下面四个基本用不到,上面四个足以 --> container: 容器的属性 jvmFlgs: JVM 容器的参数,和 Dockerfile 的 ENTRYPOINT作用相同 mainClass: 启动类限定名 args: main 方法的传入参数 ports: 容器暴露的端口,和 Dockerfile 的EXPOSE作用相同 ``` ### 构建镜像 运行maven的 ``` package jib:build ``` ### [点击查看阿里云容器配置](https://www.studyjava.cn/topic/view/0ca204db74c54635a88b5896493953e8.html)
作者:微信小助手
<p data-mpa-powered-by="yiban.io"><br></p> <section class="" style="white-space: normal;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;background-color: rgb(255, 255, 255);"> <section style="margin: 10px auto;display: flex;justify-content: center;align-items: flex-end;"> <p style="color: rgb(88, 16, 13);line-height: 1.5;text-align: center;"><strong style="color: rgb(57, 137, 31);"><img class="rich_pages" data-copyright="0" data-ratio="0.6666666666666666" data-s="300,640" data-type="jpeg" data-w="1280" src="/upload/398d99ea818be829b0277e1b59a977c8.jpg" style="letter-spacing: 0.544px;box-shadow: rgb(170, 170, 170) 0em 0em 1em 0px;visibility: visible !important;width:auto !important;max-width:100% !important;height:auto !important;"></strong><br></p> </section> </section> <p style="margin-top: 25px;margin-bottom: 15px;white-space: normal;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;background-color: rgb(255, 255, 255);line-height: 2em;text-align: center;"><span style="font-size: 20px;">扫描下方二维码<span style="color: rgb(201, 56, 28);"><strong>试读</strong></span></span></p> <p style="margin-bottom: 15px;white-space: normal;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;background-color: rgb(255, 255, 255);text-align: center;"><span style="color: rgb(57, 137, 31);"><strong><img class="" data-ratio="0.6666666666666666" data-type="png" data-w="600" src="/upload/3f6bb75371fa02356c9a40f699003d25.png" style="border-radius: 0em;width: 216.625px;visibility: visible !important;"></strong></span></p> <hr style="white-space: normal;border-style: solid;border-right-width: 0px;border-bottom-width: 0px;border-left-width: 0px;border-color: rgba(0, 0, 0, 0.0980392);transform-origin: 0px 0px 0px;transform: scale(1, 0.5);"> <p style="white-space: normal;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">多路复用其实并不是什么新技术,它的作用是在一个通讯连接的基础上可以同时进行多个请求响应处理。</span><span style="letter-spacing: 0.544px;">对于网络通讯来其实不存在这一说法,因为网络层面只负责数据传输</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="letter-spacing: 0.544px;">由于上层应用协议的制订问题,导致了很多传统服务并不能支持多路复用;</span><span style="letter-spacing: 0.544px;">如:</span><span style="letter-spacing: 0.544px;">http1.1,sqlserver和redis等等,虽然有些服务提供批量处理,但这些处理都基于一个RPS(</span><span style="letter-spacing: 0.544px;">每秒请求数</span><span style="letter-spacing: 0.544px;">)下。</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="letter-spacing: 0.544px;">下面通过图解来了解释单路和多路复用的区别。</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><img class="" data-ratio="0.6666666666666666" data-type="png" data-w="915" src="/upload/58b71116653cb12d0d759429e63b9310.null" style="margin: auto;box-sizing: inherit;border-style: none;visibility: visible !important;width:auto !important;max-width:100% !important;height:auto !important;"></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><br></p> <section class="" data-mpa-template-id="1465733" data-mpa-color="null" data-mpa-category="fav" style="font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);"> <section> <section class=""> <section style="margin-top: 1em;margin-bottom: 1em;border-bottom: 2px solid rgb(236, 68, 68);clear: both;"> <section mpa-is-content="t" style="padding-top: 3px;padding-right: 10px;padding-left: 10px;display: inline-block;color: rgb(255, 255, 255);border-top-left-radius: 3px;border-top-right-radius: 3px;height: 28px;background-color: rgb(236, 68, 68);"> <span style="font-size: 16px;">一、单路存在的问题</span> </section> <section style="margin-left: 2px;border-top: 28px solid transparent;border-right-color: rgb(206, 206, 206);border-bottom: 0px solid transparent;border-left: 15px solid rgb(206, 206, 206);display: inline-block;vertical-align: top;"></section> </section> </section> </section> </section> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">每个请求响应独占一个连接,并独占连接网络读写;这样导致连接在有大量时间被闲置无法更好地利用网络资源。</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">由于是独占读写IO,这样导致RPS处理量由必须由IO承担,IO操作起来比较损耗性能,这样在高RPS处理就出现性能问题。</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">由于不能有效的合并IO也会导致在通讯中的带宽存在浪费情况,特别对于比较小的请求数据包。</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">通讯上的延时当要持大量的RPS那就必须要有更多连接支撑,连接数增加也对资源的开销有所增加。<br></span></p> <section class="" data-mpa-template-id="1465733" data-mpa-color="null" data-mpa-category="fav" style="font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);"> <section> <section class=""> <section style="margin-top: 1em;margin-bottom: 1em;border-bottom: 2px solid rgb(236, 68, 68);clear: both;"> <section mpa-is-content="t" style="padding-top: 3px;padding-right: 10px;padding-left: 10px;display: inline-block;color: rgb(255, 255, 255);border-top-left-radius: 3px;border-top-right-radius: 3px;height: 28px;background-color: rgb(236, 68, 68);"> <span style="font-size: 16px;">二、多路复用的优点</span> </section> <section style="margin-left: 2px;border-top: 28px solid transparent;border-right-color: rgb(206, 206, 206);border-bottom: 0px solid transparent;border-left: 15px solid rgb(206, 206, 206);display: inline-block;vertical-align: top;"></section> </section> </section> </section> </section> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">多路复用可以在一个连接上同时处理多个请求响应,这样可以大大的减少连接的数量,并提高了网络的处理能力。</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">由于是共享连接不同请求响应数据包可以合并到一个IO上处理,这样可以大大降低IO的处理量,让性能表现得更出色。<br></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;"><br></span></p> <section class="" data-mpa-template-id="1465733" data-mpa-color="null" data-mpa-category="fav" style="font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);"> <section> <section class=""> <section style="margin-top: 1em;margin-bottom: 1em;border-bottom: 2px solid rgb(236, 68, 68);clear: both;"> <section mpa-is-content="t" style="padding-top: 3px;padding-right: 10px;padding-left: 10px;display: inline-block;color: rgb(255, 255, 255);border-top-left-radius: 3px;border-top-right-radius: 3px;height: 28px;background-color: rgb(236, 68, 68);"> <span style="font-size: 16px;">三、通过多路复用实现百万级RPS</span> </section> <section style="margin-left: 2px;border-top: 28px solid transparent;border-right-color: rgb(206, 206, 206);border-bottom: 0px solid transparent;border-left: 15px solid rgb(206, 206, 206);display: inline-block;vertical-align: top;"></section> </section> </section> </section> </section> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">多路复用是不是真的如此出色呢,以下在.net core上使用多路复用实现单服务百万RPS吞吐,并能达到比较低的延时性。</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">以下是测试流程: </span><img class="" data-ratio="0.6666666666666666" data-type="png" data-w="663" src="/upload/49bda6692cbf18f27cbde563a8cafdfc.null" style="margin: auto;box-sizing: inherit;border-style: none;visibility: visible !important;width:auto !important;max-width:100% !important;height:auto !important;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">由于基础通讯不具备消息包合并功能,所以在BeetleX的基础上做集成测试,主要BeetleX会自动合并消息到一个Buffer上,从而降低IO的读写。</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;"><br></span></p> <section class="" data-mpa-template-id="1465733" data-mpa-color="null" data-mpa-category="fav" style="font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);"> <section> <section class=""> <section style="margin-top: 1em;margin-bottom: 1em;border-bottom: 2px solid rgb(236, 68, 68);clear: both;"> <section mpa-is-content="t" style="padding-top: 3px;padding-right: 10px;padding-left: 10px;display: inline-block;color: rgb(255, 255, 255);border-top-left-radius: 3px;border-top-right-radius: 3px;height: 28px;background-color: rgb(236, 68, 68);"> <span style="font-size: 16px;">四、测试消息结构</span> </section> <section style="margin-left: 2px;border-top: 28px solid transparent;border-right-color: rgb(206, 206, 206);border-bottom: 0px solid transparent;border-left: 15px solid rgb(206, 206, 206);display: inline-block;vertical-align: top;"></section> </section> </section> </section> </section> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">本测试使用了Protobuf作为基础交互消息,毕竟Protobuf已经是一个二进制序列化标准了。<br></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);"><span style="color: rgb(255, 169, 0);"><strong>4.1、请求消息</strong></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><img class="" data-ratio="0.6666666666666666" data-s="300,640" data-type="png" data-w="450" src="/upload/ee9a6520ec3efb8bd95bdfee0b0bb695.null" style="width: 450px !important;visibility: visible !important;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="color: rgb(255, 169, 0);"><strong>4.2、响应消息</strong></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><img class="" data-ratio="0.6666666666666666" data-s="300,640" data-type="png" data-w="436" src="/upload/6a9da8c5d2847a76d241174ab746fffe.null" style="width: 436px !important;visibility: visible !important;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="color: rgb(255, 169, 0);"><strong>4.3、服务端处理代码</strong></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><img class="" data-ratio="0.6666666666666666" data-s="300,640" data-type="png" data-w="709" src="/upload/e928e6917e640d974b5ba5ba835cfeb7.null" style="visibility: visible !important;width:auto !important;max-width:100% !important;height:auto !important;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="color: rgb(255, 169, 0);"><strong>4.4、服务响应对象内容</strong></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><img class="" data-ratio="0.6666666666666666" data-s="300,640" data-type="png" data-w="498" src="/upload/b6de46152090aa77371a68117bc5c981.null" style="width: 498px !important;visibility: visible !important;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">接收消息后放入队列,然后由队列处理响应,设置请求相应请求时间并记录总处理消息计数。</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;"><br></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="color: rgb(255, 169, 0);"><strong><span style="font-size: 16px;">4.5、客户端请求代码</span></strong></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><img class="" data-ratio="0.6666666666666666" data-s="300,640" data-type="png" data-w="764" src="/upload/1f916387474e470a80ad7e06cc6e23d7.null" style="visibility: visible !important;width:auto !important;max-width:100% !important;height:auto !important;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="color: rgb(255, 169, 0);"><strong><br></strong></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="color: rgb(255, 169, 0);"><strong>4.6、客户端测试发起代码</strong></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><img class="" data-ratio="0.6666666666666666" data-s="300,640" data-type="png" data-w="651" src="/upload/ebcf0b52f084492d744e0121790a6197.null" style="visibility: visible !important;width:auto !important;max-width:100% !important;height:auto !important;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">整个测试开启了10个连接,在这10个连接的基础上进行请求响应复用。</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;"><br></span></p> <section class="" data-mpa-template-id="1465733" data-mpa-color="null" data-mpa-category="fav" style="font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);"> <section> <section class=""> <section style="margin-top: 1em;margin-bottom: 1em;border-bottom: 2px solid rgb(236, 68, 68);clear: both;"> <section mpa-is-content="t" style="padding-top: 3px;padding-right: 10px;padding-left: 10px;display: inline-block;color: rgb(255, 255, 255);border-top-left-radius: 3px;border-top-right-radius: 3px;height: 28px;background-color: rgb(236, 68, 68);"> <span style="font-size: 16px;">五、测试配置</span> </section> <section style="margin-left: 2px;border-top: 28px solid transparent;border-right-color: rgb(206, 206, 206);border-bottom: 0px solid transparent;border-left: 15px solid rgb(206, 206, 206);display: inline-block;vertical-align: top;"></section> </section> </section> </section> </section> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">测试环境是两台服务器,配置是阿里云上的12核服务器(对应的物理机应该是6核12线程)<br></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">服务和客户端的系统都是:Ubuntu 16.04</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">Dotnet core版本是:2.14</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;"><br></span></p> <section class="" data-mpa-template-id="1465733" data-mpa-color="null" data-mpa-category="fav" style="font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);"> <section> <section class=""> <section style="margin-top: 1em;margin-bottom: 1em;border-bottom: 2px solid rgb(236, 68, 68);clear: both;"> <section mpa-is-content="t" style="padding-top: 3px;padding-right: 10px;padding-left: 10px;display: inline-block;color: rgb(255, 255, 255);border-top-left-radius: 3px;border-top-right-radius: 3px;height: 28px;background-color: rgb(236, 68, 68);"> <span style="font-size: 16px;">六、测试结果</span> </section> <section style="margin-left: 2px;border-top: 28px solid transparent;border-right-color: rgb(206, 206, 206);border-bottom: 0px solid transparent;border-left: 15px solid rgb(206, 206, 206);display: inline-block;vertical-align: top;"></section> </section> </section> </section> </section> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><strong><span style="color: rgb(255, 169, 0);">6.1、客户端统计结果</span></strong></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><img class="" data-ratio="0.6666666666666666" data-type="png" data-w="701" src="/upload/692db1f45d8907e6d78ef94e1011058e.null" style="margin: auto;box-sizing: inherit;border-style: none;visibility: visible !important;width:auto !important;max-width:100% !important;height:auto !important;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="color: rgb(255, 169, 0);"><strong>6.2、服务端统计信息</strong></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><img class="" data-ratio="0.6666666666666666" data-type="png" data-w="671" src="/upload/4673e055f38e8fc51be6256ced12a9cd.null" style="margin: auto;box-sizing: inherit;border-style: none;visibility: visible !important;width:auto !important;max-width:100% !important;height:auto !important;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="color: rgb(255, 169, 0);"><strong>6.3、带宽统计</strong></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><img class="" data-ratio="0.6666666666666666" data-type="png" data-w="494" src="/upload/831a2eb3c1d6ff969704e0eb03888c14.null" style="margin: auto;box-sizing: inherit;border-style: none;width: 494px !important;visibility: visible !important;"><br></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;">测试使用了10个连接进行多路复用,每秒接收响应量在100W,大部分响应延时在1-3毫秒之间;</span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 16px;"><br></span></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><strong><span style="font-size: 16px;color: rgb(255, 169, 0);">6.4、测试代码</span></strong></p> <p style="margin-top: 15px;margin-bottom: 15px;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);line-height: 2em;"><span style="font-size: 11px;">https://github.com/IKende/BeetleX/blob/master/samples/MultiplexingConnectionTest.zip</span></p> <p style="white-space: normal; text-align: center;"><strong style="font-family: 微软雅黑; text-align: center; white-space: normal; color: rgb(57, 137, 31); letter-spacing: 0.544px;"><span style="font-size: 24px;">End</span></strong></p> <p style="white-space: normal;"><span style="font-size: 12px;">作者:泥水佬<br></span></p> <p style="white-space: normal;"><span style="font-size: 12px;">来源:</span></p> <p style="white-space: normal;"><span style="font-family: -apple-system-font, BlinkMacSystemFont, "Helvetica Neue", "PingFang SC", "Hiragino Sans GB", "Microsoft YaHei UI", "Microsoft YaHei", Arial, sans-serif; letter-spacing: 0.544px; text-align: right; background-color: rgb(255, 255, 255); font-size: 12px;">my.oschina.net/ikende/blog/2250622</span></p> <p style="white-space: normal;"><span style="font-size: 12px;">本文版权归作者所有</span></p> <p style="margin-right: 5px;margin-left: 5px;white-space: normal;background-color: rgb(255, 255, 255);font-family: 微软雅黑;line-height: 1.75em;letter-spacing: 0.5px;text-align: center;overflow-wrap: break-word !important;"><br></p> <p style="white-space: normal;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;background-color: rgb(255, 255, 255);line-height: 2em;text-align: center;"><span style="font-size: 14px;">长按下图二维码,即刻关注【<span style="color: rgb(241, 136, 35);"><strong>狸猫技术窝</strong></span>】</span></p> <p style="white-space: normal;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;background-color: rgb(255, 255, 255);line-height: 2em;text-align: center;"><span style="color: rgb(0, 0, 0);font-size: 14px;letter-spacing: 0.544px;">阿里、京东、美团、字节跳动</span><br></p> <p style="white-space: normal;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;background-color: rgb(255, 255, 255);line-height: 2em;text-align: center;"><span style="font-size: 14px;"><strong><span style="font-size: 13px;color: rgb(0, 0, 0);">顶尖技术专家</span></strong><span style="font-size: 13px;color: rgb(0, 0, 0);">坐镇</span></span></p> <p style="white-space: normal;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;background-color: rgb(255, 255, 255);line-height: 2em;text-align: center;"><span style="color: rgb(0, 0, 0);font-size: 14px;">为IT人打造一个 “有温度” 的技术窝!</span></p> <section class="" data-tools-id="79539" style="white-space: normal;font-family: -apple-system-font, BlinkMacSystemFont, Helvetica Neue, PingFang SC, Hiragino Sans GB, Microsoft YaHei UI, Microsoft YaHei, Arial, sans-serif;letter-spacing: 0.544px;background-color: rgb(255, 255, 255);"> <section style="margin: 10px auto;display: flex;justify-content: center;align-items: center;"> <section style="padding: 30px;background-image: url(https://mmbiz.qpic.cn/mmbiz_gif/vnOqylzBGCQHu6jrGZMlKlQlriarYFuN2jzLbPCJRlbydFZSlxHHLALHPenWJGC9WFtiaRFyMvicibKbv6nwdHibbiaw/640?wx_fmt=gif);background-position: center center;background-repeat: repeat;"> <p style="text-align: center;"><img class="rich_pages" data-copyright="0" data-ratio="0.6666666666666666" data-s="300,640" data-type="jpeg" data-w="258" src="/upload/578288c965b89f74b583291d3fc98c86.jpg" style="visibility: visible !important;width: 258px !important;"></p> </section> </section> </section> <p style="white-space: normal;"><br></p>
作者:じ☆ve宝贝
#### 特别注意:一定要用管理员权限的命令提示符 1:下载mysql-5.7.9-win32.zip 或mysql-5.7.9-winx64.zip 在官网:http://dev.mysql.com/downloads/mysql/ 中,选择以下版本的mysql下载 2:解压缩将my-default.ini改为my.ini,在my.ini文件中,加入:skip-grant-tables(不需要密码验证,直接登录) ``` #配置my.ini [mysql] default-character-set=utf8 [mysqld] port = 3306 basedir=D:\Develop\Soft\mysql-5.7.9-winx64 datadir=D:\Develop\Soft\mysql-5.7.9-winx64\data skip-grant-tables max_connections=2000 character-set-server=utf8 default-storage-engine=INNODB #密码不过期 default_password_lifetime=0 ``` 3:初始化data目录 ``` #使用cmd进入mysql的bin目录中 mysqld --initialize --user=mysql --console mysqld --install net start mysql #启动服务 net stop mysql #停止服务 sc delete mysql #删除服务 ``` 4:进入mysql控制台: ``` mysql -uroot -p ``` 5:使用mysql数据库 ``` use mysql ``` 6:修改root用户密码 ``` UPDATE user SET authentication_string= password ('root') WHERE User='root'; ``` 7:退出mysql控制台,并且注释掉my.ini中的skip-grant-tables ``` exit ``` 8:重新启动mysql ``` net start mysql #现在就可以用root和你的新密码登录了。 ``` 9:密码不过期配置:修改my.ini ``` #设置为:0 表示密码永不过期 default_password_lifetime=0 ``` 10:异常处理: 1)报错error: 'Your password has expired. To log in you must change it using a client t hat supports expired passwords.' ``` set password for 'root'@'localhost'=password('root'); ```
作者:微信小助手
<section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="padding-top: 10px;padding-right: 10px;padding-left: 10px;box-sizing: border-box;background-color: rgb(239, 239, 239);"> <span style="display: inline-block;width: 5%;line-height: 0.8;font-weight: bolder;font-size: 48px;box-sizing: border-box;"> <section style="box-sizing: border-box;"> “ </section></span> <section style="display: inline-block;vertical-align: top;float: right;width: 90%;line-height: 1.5;font-size: 15px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><span style="letter-spacing: 1px;">毫无疑问,Spring Cloud 是目前微服务架构领域的翘楚,无数的书籍博客都在讲解这个技术。</span></p> </section> <section style="clear: both;box-sizing: border-box;"></section> </section> </section> </section> </section> <p style="line-height: 1.75em;"><br></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="0.5251322751322751" data-s="300,640" src="/upload/a11dd6d9317ddb34e1904ee21416389a.png" data-type="png" data-w="756" style=""></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">不过大多数讲解还停留在对 Spring Cloud 功能使用的层面,其底层的很多原理,很多人可能并不知晓。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">因此本文将通过大量的手绘图,给大家谈谈 Spring Cloud 微服务架构的底层原理。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">实际上,Spring Cloud 是一个全家桶式的技术栈,它包含了很多组件。本文先从最核心的几个组件,<span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 28px;text-align: justify;">也就是 Eureka、Ribbon、Feign、Hystrix、Zuul </span>入手,来剖析其底层的工作原理。</span></p> <p style="line-height: normal;"><br></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="border-bottom-width: 1px;border-bottom-style: solid;border-bottom-color: black;margin-top: 0.5em;margin-bottom: 0.5em;line-height: 1.2;box-sizing: border-box;"> <section style="display: inline-block;border-bottom-width: 6px;border-bottom-style: solid;border-color: rgb(89, 89, 89);margin-bottom: -1px;font-size: 20px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;">业务场景介绍</p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">先来给大家说一个业务场景,假设咱们现在开发一个电商网站,要实现支付订单的功能。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">流程如下:</span></p> <ul class=" list-paddingleft-2" style="margin-left: 8px;margin-right: 8px;"> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">创建一个订单后,如果用户立刻支付了这个订单,我们需要将订单状态更新为“已支付”。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">扣减相应的商品库存。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">通知仓储中心,进行发货。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">给用户的这次购物增加相应的积分。</span></p></li> </ul> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">针对上述流程,我们需要有订单服务、库存服务、仓储服务、积分服务。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">整个流程的大体思路如下:</span></p> <ul class=" list-paddingleft-2" style="margin-left: 8px;margin-right: 8px;"> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">用户针对一个订单完成支付之后,就会去找订单服务,更新订单状态。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">订单服务调用库存服务,完成相应功能。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">订单服务调用仓储服务,完成相应功能。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">订单服务调用积分服务,完成相应功能。</span></p></li> </ul> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">至此,整个支付订单的业务流程结束。</span><span style="line-height: 1.6;font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">下面这张图,清晰表明了各服务间的调用过程:</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="0.5013192612137203" data-s="300,640" src="/upload/672db066be570cc30a475bcb927d769d.png" data-type="png" data-w="758" style=""></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">好!有了业务场景之后,咱们就一起来看看 Spring Cloud 微服务架构中,这几个组件如何相互协作,各自发挥的作用以及其背后的原理。</span></p> <p style="line-height: normal;"><br></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="border-bottom-width: 1px;border-bottom-style: solid;border-bottom-color: black;margin-top: 0.5em;margin-bottom: 0.5em;line-height: 1.2;box-sizing: border-box;"> <section style="display: inline-block;border-bottom-width: 6px;border-bottom-style: solid;border-color: rgb(89, 89, 89);margin-bottom: -1px;font-size: 20px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;">Spring Cloud 核心组件:Eureka</p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">咱们来考虑第一个问题:</span></strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">订单服务想要调用库存服务、仓储服务,或者积分服务,怎么调用?</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;line-height: 1.75em;margin-left: 8px;margin-right: 8px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">订单服务压根儿就不知道人家库存服务在哪台机器上啊!它就算想要发起一个请求,都不知道发送给谁,有心无力!</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;line-height: 1.75em;margin-left: 8px;margin-right: 8px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">这时候,就轮到 Spring Cloud Eureka 出场了。Eureka 是微服务架构中的注册中心,专门负责服务的注册与发现。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">咱们来看看下面的这张图,结合图来仔细剖析一下整个流程:</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="0.4357495881383855" data-s="300,640" src="/upload/a24b35c5525963cb8a9ae8e7eaa75ab9.png" data-type="png" data-w="1214" style=""></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">如上图所示,库存服务、仓储服务、积分服务中都有一个 Eureka Client 组件,这个组件专门负责将这个服务的信息注册到 Eureka Server 中。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">说白了,就是告诉 Eureka Server,自己在哪台机器上,监听着哪个端口。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">而 Eureka Server 是一个注册中心,里面有一个注册表,保存了各服务所在的机器和端口号。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">订单服务里也有一个 Eureka Client 组件,这个 Eureka Client 组件会找 Eureka Server 问一下:库存服务在哪台机器啊?监听着哪个端口啊?仓储服务呢?积分服务呢?</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">然后就可以把这些相关信息从 Eureka Server 的注册表中拉取到自己的本地缓存中来。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">这时如果订单服务想要调用库存服务,不就可以找自己本地的 Eureka Client 问一下库存服务在哪台机器?监听哪个端口吗?</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">收到响应后,紧接着就可以发送一个请求过去,调用库存服务扣减库存的那个接口!同理,如果订单服务要调用仓储服务、积分服务,也是如法炮制。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">总结一下:</span></p> <ul class=" list-paddingleft-2" style="margin-left: 8px;margin-right: 8px;"> <li><p style="text-align: justify;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">Eureka Client:</span></strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">负责将这个服务的信息注册到 Eureka Server 中。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">Eureka Server:</span></strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">注册中心,里面有一个注册表,保存了各个服务所在的机器和端口号。</span></p></li> </ul> <p style="line-height: normal;"><br></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="border-bottom-width: 1px;border-bottom-style: solid;border-bottom-color: black;margin-top: 0.5em;margin-bottom: 0.5em;line-height: 1.2;box-sizing: border-box;"> <section style="display: inline-block;border-bottom-width: 6px;border-bottom-style: solid;border-color: rgb(89, 89, 89);margin-bottom: -1px;font-size: 20px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;">Spring Cloud 核心组件:Feign</p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">现在订单服务确实知道库存服务、积分服务、仓库服务在哪里了,同时也监听着哪些端口号了。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">但是新问题又来了:</span></strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">难道订单服务要自己写一大堆代码,跟其他服务建立网络连接,然后构造一个复杂的请求,接着发送请求过去,最后对返回的响应结果再写一大堆代码来处理吗?</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">这是上述流程翻译的代码片段,咱们一起来看看,体会一下这种绝望而无助的感受!!!</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">友情提示,前方高能:</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="0.9045848822800495" data-s="300,640" src="/upload/3dd12c8061b7db02ea1935c7e5ddcb92.png" data-type="png" data-w="807" style=""></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">看完上面那一大段代码,有没有感到后背发凉、一身冷汗?实际上你进行服务间调用时,如果每次都手写代码,代码量比上面那段要多至少几倍,所以这个事压根儿就不是地球人能干的。<br></span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">既然如此,那怎么办呢?别急,Feign 早已为我们提供好了优雅的解决方案。来看看如果用 Feign 的话,你的订单服务调用库存服务的代码会变成啥样?</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="0.6293478260869565" data-s="300,640" src="/upload/ad5f65e9407f6a0d93122e066d69d289.png" data-type="png" data-w="920" style=""></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">看完上面的代码什么感觉?是不是感觉整个世界都干净了,又找到了活下去的勇气!</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">没有底层的建立连接、构造请求、解析响应的代码,直接就是用注解定义一个 Feign Client 接口,然后调用那个接口就可以了。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">人家 Feign Client 会在底层根据你的注解,跟你指定的服务建立连接、构造请求、发起请求、获取响应、解析响应,等等。这一系列脏活累活,人家 Feign 全给你干了。<br></span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">那么问题来了,Feign 是如何做到这么神奇的呢?很简单,Feign 的一个关键机制就是使用了动态代理。</span></p> <p style="line-height: normal;text-align: center;margin-left: 8px;margin-right: 8px;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="0.308890925756187" data-s="300,640" src="/upload/3f4f5ab1edb7ebf2cc435cdd2abbb8b9.png" data-type="png" data-w="1091" style="line-height: 28px;text-align: justify;white-space: normal;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">咱们一起来看看上面的图,结合图来分析:</span></p> <ul class=" list-paddingleft-2" style="margin-left: 8px;margin-right: 8px;"> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">首先,如果你对某个接口定义了 @FeignClient 注解,Feign 就会针对这个接口创建一个动态代理。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">接着你要是调用那个接口,本质就是会调用 Feign 创建的动态代理,这是核心中的核心。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">Feign的动态代理会根据你在接口上的 @RequestMapping 等注解,来动态构造出你要请求的服务的地址。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">最后针对这个地址,发起请求、解析响应。</span></p></li> </ul> <p style="line-height: normal;"><br></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="border-bottom-width: 1px;border-bottom-style: solid;border-bottom-color: black;margin-top: 0.5em;margin-bottom: 0.5em;line-height: 1.2;box-sizing: border-box;"> <section style="display: inline-block;border-bottom-width: 6px;border-bottom-style: solid;border-color: rgb(89, 89, 89);margin-bottom: -1px;font-size: 20px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;">Spring Cloud 核心组件:Ribbon</p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">说完了 Feign,还没完。现在新的问题又来了,如果人家库存服务部署在了 5 台机器上。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">如下所示:</span></p> <ul class=" list-paddingleft-2" style="margin-left: 8px;margin-right: 8px;"> <li><p style="text-align: justify;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">192.168.169:9000</span></strong></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">192.168.170:9000</span></strong></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">192.168.171:9000</span></strong></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">192.168.172:9000</span></strong></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">192.168.173:9000</span></strong></p></li> </ul> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">这下麻烦了!人家 Feign 怎么知道该请求哪台机器呢?</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">这时 Spring Cloud Ribbon 就派上用场了。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">Ribbon 就是专门解决这个问题的。它的作用是负载均衡,会帮你在每次请求时选择一台机器,均匀的把请求分发到各个机器上。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;line-height: 1.75em;margin-left: 8px;margin-right: 8px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">Ribbon 的负载均衡默认使用的最经典的 Round Robin 轮询算法。</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">这是啥?</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;line-height: 1.75em;margin-left: 8px;margin-right: 8px;"><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">简单来说,就是如果订单服务对库存服务发起 10 次请求,那就先让你请求第 1 台机器、然后是第 2 台机器、第 3 台机器、第 4 台机器、第 5 台机器,接着再来—个循环,第 1 台机器、第 2 台机器。。。以此类推。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">此外,Ribbon 是和 Feign 以及 Eureka 紧密协作,完成工作的,具体如下:</span></p> <ul class=" list-paddingleft-2" style="margin-left: 8px;margin-right: 8px;"> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">首先 Ribbon 会从 Eureka Client 里获取到对应的服务注册表,也就知道了所有的服务都部署在了哪些机器上,在监听哪些端口号。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">然后 Ribbon 就可以使用默认的 Round Robin 算法,从中选择一台机器。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">Feign 就会针对这台机器,构造并发起请求。</span></p></li> </ul> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">对上述整个过程,再来一张图,帮助大家更深刻的理解:</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="0.44352617079889806" data-s="300,640" src="/upload/866632960a530608ce78259f4face986.png" data-type="png" data-w="1452" style=""></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="border-bottom-width: 1px;border-bottom-style: solid;border-bottom-color: black;margin-top: 0.5em;margin-bottom: 0.5em;line-height: 1.2;box-sizing: border-box;"> <section style="display: inline-block;border-bottom-width: 6px;border-bottom-style: solid;border-color: rgb(89, 89, 89);margin-bottom: -1px;font-size: 20px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;">Spring Cloud 核心组件:Hystrix</p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">在微服务架构里,一个系统会有很多的服务。以本文的业务场景为例:订单服务在一个业务流程里需要调用三个服务。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">现在假设订单服务自己最多只有 100 个线程可以处理请求,然后呢,积分服务不幸的挂了,每次订单服务调用积分服务的时候,都会卡住几秒钟,然后抛出—个超时异常。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(89, 89, 89);">咱们一起来分析一下,这样会导致什么问题?</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">如果系统处于高并发的场景下,大量请求涌过来的时候,订单服务的 100 个线程都会卡在请求积分服务这块,导致订单服务没有一个线程可以处理请求。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;line-height: 1.75em;margin-left: 8px;margin-right: 8px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">然后就会导致别人请求订单服务的时候,发现订单服务也挂了,不响应任何请求了。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">上面这个,就是微服务架构中恐怖的服务雪崩问题,如下图所示:</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="0.708018154311649" data-s="300,640" src="/upload/2844c4afe3c19f06f0e4d3ff7455abd1.png" data-type="png" data-w="661" style=""></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">如上图,这么多服务互相调用,要是不做任何保护的话,某一个服务挂了,就会引起连锁反应,导致别的服务也挂。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">比如积分服务挂了,会导致订单服务的线程全部卡在请求积分服务这里,没有一个线程可以工作,瞬间导致订单服务也挂了,别人请求订单服务全部会卡住,无法响应。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">但是我们思考一下,就算积分服务挂了,订单服务也可以不用挂啊!为什么?</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;line-height: 1.75em;margin-left: 8px;margin-right: 8px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">我们结合业务来看:支付订单的时候,只要把库存扣减了,然后通知仓库发货就 OK 了。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;line-height: 1.75em;margin-left: 8px;margin-right: 8px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">如果积分服务挂了,大不了等它恢复之后,慢慢人肉手工恢复数据!为啥一定要因为一个积分服务挂了,就直接导致订单服务也挂了呢?不可以接受!</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">现在问题分析完了,如何解决?<span style="letter-spacing: 1px;color: rgb(89, 89, 89);font-size: 15px;line-height: 1.6;">这时就轮到 Hystrix 闪亮登场了。Hystrix 是隔离、熔断以及降级的一个框架。啥意思呢?</span></span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">说白了,Hystrix 会搞很多个小小的线程池,比如订单服务请求库存服务是一个线程池,请求仓储服务是一个线程池,请求积分服务是一个线程池。每个线程池里的线程就仅仅用于请求那个服务。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">打个比方:</span></strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">现在很不幸,积分服务挂了,会咋样?<span style="letter-spacing: 1px;color: rgb(89, 89, 89);font-size: 15px;line-height: 1.6;">当然会导致订单服务里那个用来调用积分服务的线程都卡死不能工作了啊!</span></span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="line-height: 1.6;font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">但由于订单服务调用库存服务、仓储服务的这两个线程池都是正常工作的,所以这两个服务不会受到任何影响。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">这个时候如果别人请求订单服务,订单服务还是可以正常调用库存服务扣减库存,调用仓储服务通知发货。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">只不过调用积分服务的时候,每次都会报错。但是如果积分服务都挂了,每次调用都要去卡住几秒钟干啥呢?有意义吗?当然没有!</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">所以我们直接对积分服务熔断不就得了,比如在 5 分钟内请求积分服务直接就返回了,不要去走网络请求卡住几秒钟,这个过程,就是所谓的熔断!</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">那人家又说,兄弟,积分服务挂了你就熔断,好歹你干点儿什么啊!别啥都不干就直接返回啊?</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">没问题,咱们就来个降级:每次调用积分服务,你就在数据库里记录一条消息,说给某某用户增加了多少积分,因为积分服务挂了,导致没增加成功!</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">这样等积分服务恢复了,你可以根据这些记录手工加一下积分。这个过程,就是所谓的降级。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">为帮助大家更直观的理解,接下来用一张图,梳理一下 Hystrix 隔离、熔断和降级的全流程:</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="0.7448107448107448" data-s="300,640" src="/upload/75fe07dab278c2ccc7e734556406d98f.png" data-type="png" data-w="819" style=""></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="border-bottom-width: 1px;border-bottom-style: solid;border-bottom-color: black;margin-top: 0.5em;margin-bottom: 0.5em;line-height: 1.2;box-sizing: border-box;"> <section style="display: inline-block;border-bottom-width: 6px;border-bottom-style: solid;border-color: rgb(89, 89, 89);margin-bottom: -1px;font-size: 20px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;">Spring Cloud 核心组件:Zuul</p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">说完了 Hystrix,接着给大家说说最后一个组件:Zuul,也就是微服务网关。这个组件是负责网络路由的。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">不懂网络路由?行,那我给你说说,如果没有 Zuul 的日常工作会怎样?</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">假设你后台部署了几百个服务,现在有个前端兄弟,人家请求是直接从浏览器那儿发过来的。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">打个比方:</span></strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">人家要请求一下库存服务,你难道还让人家记着这服务的名字叫做 inventory-service?部署在 5 台机器上?</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">就算人家肯记住这一个,你后台可有几百个服务的名称和地址呢?难不成人家请求一个,就得记住一个?你要这样玩儿,那真是友谊的小船,说翻就翻!</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">上面这种情况,压根儿是不现实的。所以一般微服务架构中都必然会设计一个网关在里面。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">像 Android、iOS、PC 前端、微信小程序、H5 等等,不用去关心后端有几百个服务,就知道有一个网关,所有请求都往网关走,网关会根据请求中的一些特征,将请求转发给后端的各个服务。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">而且有一个网关之后,还有很多好处,比如可以做统一的降级、限流、认证授权、安全,等等。</span></p> <p style="line-height: normal;"><br></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="border-bottom-width: 1px;border-bottom-style: solid;border-bottom-color: black;margin-top: 0.5em;margin-bottom: 0.5em;line-height: 1.2;box-sizing: border-box;"> <section style="display: inline-block;border-bottom-width: 6px;border-bottom-style: solid;border-color: rgb(89, 89, 89);margin-bottom: -1px;font-size: 20px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;">总结</p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">最后再来总结一下,上述几个 Spring Cloud 核心组件,在微服务架构中,分别扮演的角色:</span></p> <ul class=" list-paddingleft-2" style="margin-left: 8px;margin-right: 8px;"> <li><p style="text-align: justify;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">Eureka:</span></strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">各个服务启动时,Eureka Client 都会将服务注册到 Eureka Server,并且 Eureka Client 还可以反过来从 Eureka Server 拉取注册表,从而知道其他服务在哪里。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">Ribbon:</span></strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">服务间发起请求的时候,基于 Ribbon 做负载均衡,从一个服务的多台机器中选择一台。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">Feign:</span></strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">基于 Feign 的动态代理机制,根据注解和选择的机器,拼接请求 URL 地址,发起请求。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">Hystrix:</span></strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">发起请求是通过 Hystrix 的线程池来走的,不同的服务走不同的线程池,实现了不同服务调用的隔离,避免了服务雪崩的问题。</span></p></li> <li><p style="text-align: justify;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">Zuul:</span></strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">如果前端、移动端要调用后端系统,统一从 Zuul 网关进入,由 Zuul 网关转发请求给对应的服务。</span></p></li> </ul> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">以上就是我们通过一个电商业务场景,阐述了 Spring Cloud 微服务架构几个核心组件的底层原理。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">文字总结还不够直观?没问题!我们将 Spring Cloud 的 5 个核心组件通过一张图串联起来,再来直观的感受一下其底层的架构原理:</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="0.5658042744656918" data-s="300,640" src="/upload/f1cc8e176f5f9f9d68ec26cc55b4a0de.png" data-type="png" data-w="889" style=""></p> <p style="white-space: normal;text-align: justify;line-height: 1.75em;"><span style="color: rgb(89, 89, 89);letter-spacing: 1px;"><em><span style="font-size: 14px;">作者:</span></em></span><span style="color: rgb(89, 89, 89);letter-spacing: 1px;font-size: 14px;"><em>中华石杉</em></span></p> <p style="white-space: normal;text-align: justify;line-height: 1.75em;"><span style="color: rgb(89, 89, 89);letter-spacing: 1px;"><em><span style="font-size: 14px;">编辑:陶家龙、孙淑娟</span></em></span><br></p> <p style="text-align: justify;line-height: 1.75em;"><span style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;"><em>出处:转载自微信公众号:石杉的架构笔记(ID:shishan100)</em></span></p> <p style="line-height: normal;"><br></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="text-align: center;box-sizing: border-box;"> <section style="transform: rotate(-45deg);-webkit-transform: rotate(-45deg);-moz-transform: rotate(-45deg);-o-transform: rotate(-45deg);display: inline-block;vertical-align: middle;box-sizing: border-box;"> <section style="width: 3em;height: 3em;border-left-width: 5px;border-left-style: solid;border-left-color: rgb(89, 89, 89);border-top-width: 5px;border-top-style: solid;border-top-color: rgb(89, 89, 89);border-radius: 5em 0px 0px;box-sizing: border-box;"></section> </section> <section style="width: 7em;height: 7em;display: inline-block;vertical-align: middle;border-radius: 100%;margin-right: -2.18em;margin-left: -2.2em;box-sizing: border-box;background-color: rgb(255, 255, 255);"> <section style="width: 6em;height: 6em;margin: 0.5em auto;border-radius: 100%;box-sizing: border-box;background-image: url("https://mmbiz.qpic.cn/mmbiz/MOwlO0INfQpWQFWiaVTLtKCtrjXukEgJZd7mzjX68gwFFfAMkicv7bj0IJcMeuoiax8x8UKC7iaGlbTRcrzelZLcrA/640?wx_fmt=other");background-size: cover;background-position: 50% 50%;background-repeat: no-repeat;"> <section style="width: 100%;height: 100%;overflow: hidden;box-sizing: border-box;"> <img data-ratio="0.8607595" src="/upload/13133ffd479eee410e16a9098f03c626.png" data-type="png" data-w="158" style="width: 100%;height: 100%;opacity: 0;box-sizing: border-box;"> </section> </section> </section> <section style="display: inline-block;vertical-align: middle;transform: rotate(45deg);-webkit-transform: rotate(45deg);-moz-transform: rotate(45deg);-o-transform: rotate(45deg);box-sizing: border-box;"> <section style="width: 3em;height: 3em;border-right-width: 5px;border-right-style: solid;border-right-color: rgb(89, 89, 89);border-top-width: 5px;border-top-style: solid;border-top-color: rgb(89, 89, 89);border-radius: 0px 5em 0px 0px;box-sizing: border-box;"></section> </section> <section style="width: 100%;height: 0px;border-top-width: 2px;border-top-style: solid;border-top-color: rgb(89, 89, 89);margin-top: -3.5em;margin-bottom: 3.5em;box-sizing: border-box;"></section> </section> </section> </section> <p style="text-align: justify;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;">中华石杉:十余年 BAT 架构经验,一线互联网公司技术总监。带领上百人团队开发过多个亿级流量高并发系统。现将多年工作中积累下的研究手稿、经验总结整理成文,倾囊相授。微信公众号:石杉的架构笔记(ID:shishan100)。</span></p> <p style="text-align: center;"><img class="" data-copyright="0" data-ratio="0.3939393939393939" src="/upload/70dc65115d35e7d4c78cd84ded7bacac.gif" data-type="gif" data-w="660" style=""></p> <p style="text-align: center;"> <mp-miniprogram class="miniprogram_element" data-miniprogram-appid="wxc58e6e4759a08388" data-miniprogram-path="pages/bkdetail?id=125" data-miniprogram-nickname="51CTO播客" data-miniprogram-avatar="http://mmbiz.qpic.cn/mmbiz_png/M3DmwKCWDnP2VudjoA84HWJSg9Ige9Gfy7AKjdzNgmUkbYqIEIagHNoAGmSBibBxEMRNEjOJJArsoFchiakzmtxg/640?wx_fmt=png&wxfrom=200" data-miniprogram-title="51CTO播客,随时随地,碎片化学习" data-miniprogram-imageurl="http://mmbiz.qpic.cn/mmbiz_jpg/MOwlO0INfQpWQFWiaVTLtKCtrjXukEgJZqFAUxaJOpqj6EfOW4NjqOJDZXHBJSn3sH11J1sFNCKHUM0d7t4qUZg/0?wx_fmt=jpeg"></mp-miniprogram></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 0.5em;margin-bottom: 0.5em;box-sizing: border-box;"> <section style="font-size: 15px;border-style: solid;border-width: 0px 0px 1px;color: rgb(89, 89, 89);border-bottom-color: rgba(215, 215, 215, 0.960784);box-sizing: border-box;"> <p style="box-sizing: border-box;"><span style="letter-spacing: 1px;"><strong>精彩文章推荐:</strong></span></p> </section> </section> </section> </section> <p style="text-align: justify;line-height: 2em;"><a href="http://mp.weixin.qq.com/s?__biz=MjM5ODI5Njc2MA==&mid=2655819902&idx=1&sn=63524a042ff8604226803b9af55ff484&chksm=bd74d5a98a035cbfd73f22185df803c2aa18fdd87b8fff71f6f31823fb4d3f81bcaf9273c3eb&scene=21#wechat_redirect" target="_blank" style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;text-decoration: none;"><span style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;">老司机避坑指南:如何快速搞定微服务架构?</span></a><br></p> <p style="text-align: justify;line-height: 2em;"><a href="http://mp.weixin.qq.com/s?__biz=MjM5ODI5Njc2MA==&mid=2655819419&idx=1&sn=e73c6259e380b124cf08041ab857b87d&chksm=bd74db4c8a03525af09e2cd9c2a1143368c11fa337c5f450889519bfbe2f0e8d017fa497d0db&scene=21#wechat_redirect" target="_blank" style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;text-decoration: none;"><span style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;">给你一份超详细Spring Boot知识清单</span></a><br></p> <p style="text-align: justify;line-height: 2em;"><a href="http://mp.weixin.qq.com/s?__biz=MjM5ODI5Njc2MA==&mid=2655820082&idx=1&sn=d4b67111a15195f703c3ca879dd86ae9&chksm=bd74d4e58a035df3a8c7ec338e627f439d9129f745c453c1cafbcd42cd30b1c04cfbf8721e04&scene=21#wechat_redirect" target="_blank" style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;text-decoration: none;"><span style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;">资深大牛吐血总结:如何成为一名合格的云架构师?</span></a><br></p>
作者:微信小助手
<p style="max-width: 100%;min-height: 1em;color: rgb(51, 51, 51);font-family: -apple-system-font, system-ui, "Helvetica Neue", "PingFang SC", "Hiragino Sans GB", "Microsoft YaHei UI", "Microsoft YaHei", Arial, sans-serif;font-size: 17px;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);text-align: center;box-sizing: border-box !important;overflow-wrap: break-word !important;"><span style="max-width: 100%;color: rgb(255, 0, 0);font-size: 14px;box-sizing: border-box !important;overflow-wrap: break-word !important;"><span style="max-width: 100%;color: rgb(255, 41, 65);line-height: 22.4px;box-sizing: border-box !important;overflow-wrap: break-word !important;">(点击</span><span style="max-width: 100%;line-height: 22.4px;color: rgb(0, 128, 255);box-sizing: border-box !important;overflow-wrap: break-word !important;">上方公众号</span><span style="max-width: 100%;color: rgb(255, 41, 65);line-height: 22.4px;box-sizing: border-box !important;overflow-wrap: break-word !important;">,可快速关注)</span></span></p> <p style="max-width: 100%;min-height: 1em;color: rgb(51, 51, 51);font-family: -apple-system-font, system-ui, "Helvetica Neue", "PingFang SC", "Hiragino Sans GB", "Microsoft YaHei UI", "Microsoft YaHei", Arial, sans-serif;font-size: 17px;letter-spacing: 0.544px;text-align: justify;white-space: normal;background-color: rgb(255, 255, 255);box-sizing: border-box !important;overflow-wrap: break-word !important;"><br style="max-width: 100%;box-sizing: border-box !important;overflow-wrap: break-word !important;"></p> <blockquote style="max-width: 100%;color: rgb(51, 51, 51);font-family: -apple-system-font, system-ui, "Helvetica Neue", "PingFang SC", "Hiragino Sans GB", "Microsoft YaHei UI", "Microsoft YaHei", Arial, sans-serif;font-size: 17px;letter-spacing: 0.544px;text-align: justify;white-space: normal;background-color: rgb(255, 255, 255);box-sizing: border-box !important;overflow-wrap: break-word !important;"> <p style="max-width: 100%;min-height: 1em;text-align: left;box-sizing: border-box !important;overflow-wrap: break-word !important;"><span style="max-width: 100%;font-size: 14px;box-sizing: border-box !important;overflow-wrap: break-word !important;">来源:Rainstorm ,</span></p> <p style="max-width: 100%;min-height: 1em;text-align: left;box-sizing: border-box !important;overflow-wrap: break-word !important;"><span style="max-width: 100%;font-size: 14px;box-sizing: border-box !important;overflow-wrap: break-word !important;">github.com/c-rainstorm/blog/blob/master/devops/本机搭建三节点k8s集群.md</span></p> </blockquote> <p><br></p> <p>毕业设计题目是写一个基于微服务的高可用应用系统。微服务的部署使用 docker + k8s,所以前提是要有一个 k8s 集群。搭建过程中遇到了一些坑,把整个流程 记录下来一是回顾搭建过程,二是希望能尽可能的帮助后来的 k8s beginners 少走些弯路。</p> <p><br></p> <p>本文的侧重点在实际操作,k8s 组件基本概念相关的信息请参考 <span style="color: rgb(255, 104, 39);text-decoration: underline;">k8s 官方文档</span> 或 <span style="color: rgb(255, 104, 39);text-decoration: underline;">Kubernetes 指南 – by feiskyer</span></p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">https://kubernetes.io/docs/concepts/</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">https://github.com/feiskyer/kubernetes-handbook</span></p> </blockquote> <p><br></p> <p>如果对于本文有什么建议、意见及疑问,欢迎提 issue,或直接发邮件交流(邮箱很容易能找到,不再提供)。</p> <p><br></p> <p><strong><span style="color: rgb(255, 76, 65);">环境依赖</span></strong></p> <p><br></p> <p>本文假设读者会使用 Linux 命令行。</p> <p><br></p> <p>k8s 的镜像源在墙外,所以需要读者掌握科学上网的技能。这个部分不属于本文的描述范围,我使用的方案是 Shadowsocks + Privoxy,有需要可以跟我交流。</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">- Docker : 1.13.1</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">- kube* : 1.10</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">- flannel : 0.10.0</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">- virtualBox: 5.2.8</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">- OS : ubuntu-16.04.4-server-amd64.iso</span></p> </blockquote> <p><br></p> <p>virtualBox 的版本不太重要,Docker 和 kube* 的版本最好使用 k8s 推荐的版本,否则可能会有问题,推荐的 Docker 版本可以在版本的 release 文档中查看。OS 的版本不同,后面的一些配置的方法会略有不同。</p> <p><br></p> <p><strong><span style="color: rgb(255, 76, 65);">搭建目标</span></strong></p> <p><br></p> <p style="text-align: center;"><img class="" data-ratio="0.21870047543581617" data-s="300,640" src="/upload/db018bab4bb42f7a6a7324bc69333475.png" data-type="png" data-w="1262" style=""></p> <p><br></p> <p>flannel.1 是 每台机器上的一个 VNI,通过 ifconfig 命令可以查看该接口的信息。</p> <p><br></p> <p>从任意一台机器 ping 另外两台机器 flanel.1 的 IP 能通就算集群搭建成功。</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"># from master<br></span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">$ ping 10.244.1.0</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">PING 10.244.1.0 (10.244.1.0) 56(84) bytes of data.</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">64 bytes from 10.244.1.0: icmp_seq=1 ttl=64 time=0.659 ms</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">64 bytes from 10.244.1.0: icmp_seq=2 ttl=64 time=0.478 ms</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">64 bytes from 10.244.1.0: icmp_seq=3 ttl=64 time=0.613 ms</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">^C</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">--- 10.244.1.0 ping statistics ---</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">3 packets transmitted, 3 received, 0% packet loss, time 1999ms</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">rtt min/avg/max/mdev = 0.478/0.583/0.659/0.079 ms</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"> </span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">$ ping 10.244.2.0</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">PING 10.244.2.0 (10.244.2.0) 56(84) bytes of data.</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">64 bytes from 10.244.2.0: icmp_seq=1 ttl=64 time=0.459 ms</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">64 bytes from 10.244.2.0: icmp_seq=2 ttl=64 time=0.504 ms</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">64 bytes from 10.244.2.0: icmp_seq=3 ttl=64 time=0.553 ms</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">^C</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">--- 10.244.2.0 ping statistics ---</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">3 packets transmitted, 3 received, 0% packet loss, time 2058ms</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">rtt min/avg/max/mdev = 0.459/0.505/0.553/0.042 ms</span></p> </blockquote> <p><br></p> <p><strong><span style="color: rgb(255, 76, 65);">k8s 个组件之间的关系</span></strong></p> <p><br></p> <p style="text-align: center;"><img class="" data-ratio="0.4326171875" src="/upload/44e1036b9cb490082b717ea9c393dc27.png" data-type="png" data-w="1024"></p> <p><br></p> <p>其实这个没必要多说,<span style="color: rgb(255, 104, 39);text-decoration: underline;">Kubernetes Components</span> 或 <span style="color: rgb(255, 104, 39);text-decoration: underline;">Kubernetes 核心组件 – by feiskyer</span> 讲的已经非常好了,这里提到只是强调一下这部分的重要性。</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">https://kubernetes.io/docs/concepts/overview/components/</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">https://kubernetes.feisky.xyz/zh/components/</span></p> </blockquote> <p><br></p> <p><strong><span style="color: rgb(255, 76, 65);">k8s Pod 网络模型简介</span></strong></p> <p><br></p> <p style="text-align: center;"><img class="aligncenter size-large wp-image-30493" data-ratio="0.6884765625" src="/upload/520cd7edbb6dc7469a9231c9eafc28e9.png" data-type="png" data-w="1024" style="border-width: 0px;border-style: initial;border-color: initial;font-size: 0px;color: transparent;vertical-align: middle;text-align: center;font-family: "Microsoft YaHei", 宋体, "Myriad Pro", Lato, "Helvetica Neue", Helvetica, Arial, sans-serif;white-space: normal;background-color: rgb(255, 255, 255);" title="k8s-net-model"></p> <p><br></p> <p>图片来源:<span style="text-decoration: underline;color: rgb(255, 104, 39);">一次Flannel和Docker网络不通定位问题</span></p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">http://www.cnblogs.com/ericnie/p/8028681.html</span></p> </blockquote> <p><br></p> <p>为了省事,我直接引用了别人画的模型图。对于本次搭建的集群模型简单描述如下:</p> <p><br></p> <p style="text-align: center;"><img class="aligncenter size-full wp-image-30492" data-ratio="0.20887445887445888" src="/upload/3ae807917654bed3112ffe01024ab3a4.png" data-type="png" data-w="924" style="border-width: 0px;border-style: initial;border-color: initial;font-size: 0px;color: transparent;vertical-align: middle;text-align: center;margin: 0px;top: 0px;left: 0px;right: 0px;bottom: 0px;" title="Snipaste_2018-11-19_12-43-34"></p> <p><br></p> <ul class=" list-paddingleft-2" style="list-style-type: disc;"> <li><p>cni0 和 flannel.1 都是 VNI,由 flannel 组件创建,集群搭建完成后可以通过 ifconfig 进行查看。</p></li> <li><p>flannel 组件 在每台机器上运行了一个 flanneld,它是用来转发流量,解决主机间通信的问题。</p></li> <li><p>enp0s8 是 virtualBox 的 Host-Only 适配器。</p></li> </ul> <p><br></p> <p><strong><span style="color: rgb(255, 76, 65);">配置流程</span></strong></p> <p><br></p> <p><span style="color: rgb(123, 12, 0);"><strong>使用 virtualBox 创建三台虚拟机</strong></span></p> <p><br></p> <p>virtualBox 安装比较简单,不再介绍,GUI 工具用起来也很方便,这部分只介绍我认为需要提示的部分。</p> <p><br></p> <ol class=" list-paddingleft-2" style="list-style-type: decimal;"> <li><p>内存推荐 2048M, CPU 推荐 2个</p></li> <li><p>默认只有一个 NAT 适配器,添加一个 Host-Only Adapter。NAT 适配器是虚拟机用来访问互联网的,Host-Only 适配器是用来虚拟机之间通信的。</p></li> <li><p>以 Normal Start 方式启动虚拟机安装完系统以后,因为是 server 版镜像,所以没有图形界面,直接使用用户名密码登录即可。</p></li> <li><p>修改配置,enp0s8 使用静态 IP。配置请参考 <span style="text-decoration: underline;color: rgb(255, 104, 39);">SSH between Mac OS X host and Virtual Box guest</span>。注意配置时将其中的网络接口名改成你自己的 Host-Only Adapter 对应的接口。</p></li> <li><p>一台虚拟机创建完成以后可以使用 clone 方法复制出两台节点出来,注意 clone 时为新机器的网卡重新初始化 MAC 地址。</p></li> <li><p>三台虚拟机的静态 IP 都配置好以后就可以使用 ssh 在本地主机的终端上操作三台虚机了。虚机使用 Headless Start 模式启动</p></li> </ol> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">https://gist.github.com/c-rainstorm/1bbd44b388acd35ca6eaf07d1fbd9bc7</span></p> </blockquote> <p><br></p> <p><strong><span style="color: rgb(123, 12, 0);">安装 Docker</span></strong></p> <p><br></p> <p><strong>三台都装</strong></p> <p><br></p> <p>当前 Ubuntu 的 docker 版本刚好合适,所以可以直接安装,但是有必要提前查看一下 docker 版本,以免装错。</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"># apt search docker.io</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">apt-get update</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">apt-get install -y docker.io</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">systemctl enable docker</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">systemctl start docker</span></p> </blockquote> <p><br></p> <p><strong>docker 配置代理</strong>。镜像源在墙外,docker pull image 需要代理</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">mkdir -p /etc/systemd/system/docker.service.d</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"> </span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">cat <<EOF >/etc/systemd/system/docker.service.d/http-proxy.conf</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">[Service]</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">Environment="HTTP_PROXY=https://192.168.99.1:8118/"</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">Environment="HTTPS_PROXY=https://192.168.99.1:8118/"</span></p> </blockquote> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">Environment="NO_PROXY=localhost,127.0.0.1,localaddress,.localdomain.com"</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">EOF</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"> </span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">systemctl daemon-reload</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">systemctl restart docker</span></p> </blockquote> <p><br></p> <p><strong>测试配置</strong></p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">docker info | grep Proxy # 有输出说明配置成功</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"> </span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">docker pull gcr.io/google-containers/busybox:1.27 # pull 成功代表工作正常。</span></p> </blockquote> <p><br></p> <p>Google 镜像库</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">https://console.cloud.google.com/gcr/images/google-containers/GLOBAL?project=google-containers</span></p> </blockquote> <p><br></p> <p><span style="color: rgb(123, 12, 0);"><strong>安装 kube* 组件</strong></span></p> <p><br></p> <p>三台都装</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">apt-get update && apt-get install -y apt-transport-https</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">curl -s https://packages.cloud.google.com/apt/doc/apt-key.gpg | apt-key add -</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">cat <<EOF >/etc/apt/sources.list.d/kubernetes.list</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">deb http://apt.kubernetes.io/ kubernetes-xenial main</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">EOF</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">apt-get update</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">apt-get install -y kubelet kubeadm kubectl</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">systemctl enable kubelet</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">systemctl start kubelet</span></p> </blockquote> <p><br></p> <p>Master 节点配置 cgroup driver</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">docker info | grep -i cgroup # 一般是 cgroupfs</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">cat /etc/systemd/system/kubelet.service.d/10-kubeadm.conf # --cgroup-driver 对应值默认是 systemd</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"> </span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">#两个值不一致的话使用以下命令修改</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">sed -i "s/cgroup-driver=systemd/cgroup-driver=cgroupfs/g" /etc/systemd/system/kubelet.service.d/10-kubeadm.conf</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"> </span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">#重启 kubelet</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">systemctl daemon-reload</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">systemctl restart kubelet</span></p> </blockquote> <p><br></p> <p>curl 访问的是墙外的网站,无法访问的话可以配置系统代理。</p> <p>http://192.168.99.1:8118/ 是我本地使用的 HTTP 代理,Privoxy 监听 8118 端口,按照实际情况修改该地址。</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"># 全局代理,不推荐</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">cat <<EOF >>/etc/environment</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">http_proxy="http://192.168.99.1:8118/"</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">https_proxy="http://192.168.99.1:8118/"</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">no_proxy="master, node1, node2, 192.168.99.1"</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">EOF</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">source /etc/environment</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"> </span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">#当前 shell,推荐</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">export http_proxy="http://192.168.99.1:8118/"</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">export https_proxy="http://192.168.99.1:8118/"</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">export no_proxy="master, node1, node2, 192.168.99.1"</span></p> </blockquote> <p><br></p> <p>apt 使用代理</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">cat <<EOF >>/etc/apt/apt.conf</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">Acquire::http::Proxy "http://192.168.99.1:8118/";</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">Acquire::https::Proxy "https://192.168.99.1:8118/";</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">EOF</span></p> </blockquote> <p><br></p> <p><span style="color: rgb(123, 12, 0);"><strong>搭建集群</strong></span></p> <p><br></p> <p><strong>禁用交换区</strong></p> <p><br></p> <p>k8s 文档明确要求的。</p> <p><br></p> <p>三台都禁用</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">swapoff -a</span></p> </blockquote> <p><br></p> <p><strong>初始化 master 节点</strong></p> <p><br></p> <p>初始化过程会访问墙外网站,如果 init 不能顺利执行,请配置全局代理</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">kubeadm init --apiserver-advertise-address=192.168.99.200 --pod-network-cidr=10.244.0.0/16</span></p> </blockquote> <p><br></p> <ol class=" list-paddingleft-2" style="list-style-type: decimal;"> <li><p>--apiserver-advertise-address=192.168.99.200 绑定 apiserver 到 master 节点的 Host-Only 适配器的地址,默认是绑到 NAT 的地址上,这样其他机器是永远也访问不到的。</p></li> <li><p>--pod-network-cidr=10.244.0.0/16 指定 pod 网络地址空间,我们使用 flannel 组件必须使用这个空间。</p></li> <li><p>kubeadm 的完整参考手册 kubeadm reference guide</p></li> <li><p>推荐保存最后输出的 join 命令到文件(以免忘记或找不到了),方便添加节点到集群。如果忘了也找不到输出了,网上有方法生成哈希值,请自行查找。</p></li> </ol> <p><br></p> <p>配置 kubectl 访问集群</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"># root user</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">export KUBECONFIG=/etc/kubernetes/admin.conf</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"> </span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"># non-root user</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">mkdir -p $HOME/.kube</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">sudo cp -i /etc/kubernetes/admin.conf $HOME/.kube/config</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">sudo chown $(id -u):$(id -g) $HOME/.kube/config</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"> </span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"># 从本地主机操作,需要在本地安装 kubectl 客户端</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">mkdir -p ~/.kube</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"> </span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">scp <username>@192.168.99.200:/home/<username>/.kube/config ~/.kube/config # 从 master 复制配置文件到本地</span></p> </blockquote> <p><br></p> <p>测试配置</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">kubectl cluster-info #有正常输出即可</span></p> </blockquote> <p><br></p> <p><strong>安装 flannel 网络</strong></p> <p><br></p> <p>flannel 默认的监听接口是 NAT 适配器的接口,我们需要的是 Host-Only 适配器的接口,所以需要修改 kube-flannel.yml 文件</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">wget https://raw.githubusercontent.com/coreos/flannel/master/Documentation/kube-flannel.yml</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"> </span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">给 /opt/bin/flanneld 命令添加 --iface="enp0s8" 参数 # enp0s8 是 Host-Only 适配器对应的接口</span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);"> </span></p> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">kubectl apply -f kube-flannel.yml</span></p> </blockquote> <p><br></p> <p>测试配置</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">kubectl get pods --all-namespaces -o wide # 稍等一会,下载镜像需要一定时间,最后应该显示 flannel pods 是 Running 状态, kube-dns 也是 Running 状态</span></p> </blockquote> <p><br></p> <p>消除 master 隔离。默认 master 上不调度 pods,要允许另外的 pods 在 master 上运行请执行该命令</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">kubectl taint nodes --all node-role.kubernetes.io/master-</span></p> </blockquote> <p><br></p> <p>添加节点到集群</p> <p><br></p> <p>在节点上执行 kubeadm init 最后输出的 join 命令</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">kubeadm join --token <token> <master-ip>:<master-port> --discovery-token-ca-cert-hash sha256:<hash></span></p> </blockquote> <p><br></p> <p><strong><span style="color: rgb(255, 76, 65);">最后</span></strong></p> <p><br></p> <p>feiskyer 为该搭建过程写了<span style="color: rgb(255, 104, 39);text-decoration: underline;">自动化脚本</span>,有兴趣可以尝试一下。</p> <p><br></p> <blockquote> <p><span style="font-size: 12px;color: rgb(136, 136, 136);">https://github.com/feiskyer/ops</span></p> </blockquote> <p><br></p> <p><strong><span style="color: rgb(255, 76, 65);">参考</span></strong></p> <p><br></p> <ol class=" list-paddingleft-2" style="list-style-type: decimal;"> <li><p>k8s 官方文档</p><p>https://kubernetes.io/docs/concepts/<br></p></li> <li><p>Kubernetes 指南 – by feiskyer</p><p>https://github.com/feiskyer/kubernetes-handbook<br></p></li> <li><p>一次Flannel和Docker网络不通定位问题</p><p>http://www.cnblogs.com/ericnie/p/8028681.html<br></p></li> <li><p>coreos/flannel – README</p><p>https://github.com/coreos/flannel/blob/master/README.md<br></p></li> <li><p>Installing kubeadm</p><p>https://kubernetes.io/docs/setup/independent/install-kubeadm/<br></p></li> <li><p>Docker Ubuntu Behind Proxy</p><p>https://stackoverflow.com/questions/26550360/docker-ubuntu-behind-proxy?utm_medium=organic&utm_source=google_rich_qa&utm_campaign=google_rich_qa<br></p></li> <li><p>Using kubeadm to Create a Cluster</p><p>https://kubernetes.io/docs/setup/independent/create-cluster-kubeadm/<br></p></li> </ol> <p><br></p> <p style="max-width: 100%;min-height: 1em;color: rgb(51, 51, 51);font-family: -apple-system-font, system-ui, "Helvetica Neue", "PingFang SC", "Hiragino Sans GB", "Microsoft YaHei UI", "Microsoft YaHei", Arial, sans-serif;font-size: 17px;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);text-align: center;box-sizing: border-box !important;overflow-wrap: break-word !important;"><span style="max-width: 100%;font-size: 14px;color: rgb(255, 169, 0);box-sizing: border-box !important;overflow-wrap: break-word !important;">看完本文有收获?请转发分享给更多人</span></p> <p style="max-width: 100%;min-height: 1em;color: rgb(51, 51, 51);font-family: -apple-system-font, system-ui, "Helvetica Neue", "PingFang SC", "Hiragino Sans GB", "Microsoft YaHei UI", "Microsoft YaHei", Arial, sans-serif;font-size: 17px;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);text-align: center;box-sizing: border-box !important;overflow-wrap: break-word !important;"><strong style="max-width: 100%;color: rgb(255, 169, 0);box-sizing: border-box !important;overflow-wrap: break-word !important;">关注「ImportNew」,提升Java技能</strong></p> <p style="max-width: 100%;min-height: 1em;color: rgb(51, 51, 51);font-family: -apple-system-font, system-ui, "Helvetica Neue", "PingFang SC", "Hiragino Sans GB", "Microsoft YaHei UI", "Microsoft YaHei", Arial, sans-serif;font-size: 17px;letter-spacing: 0.544px;white-space: normal;background-color: rgb(255, 255, 255);text-align: center;box-sizing: border-box !important;overflow-wrap: break-word !important;"><img class="" data-ratio="0.9166666666666666" data-s="300,640" data-type="png" data-w="600" width="auto" src="/upload/899866149276fa5fddb73c61ae04be64.png" style="box-sizing: border-box !important;overflow-wrap: break-word !important;visibility: visible !important;width: 600px !important;"></p>
作者:じ☆ve宝贝
Windows:[https://raw.githubusercontent.com/getlantern/lantern-binaries/master/lantern-installer-beta.exe](https://raw.githubusercontent.com/getlantern/lantern-binaries/master/lantern-installer-beta.exe) OSX 10.8 and above:[https://raw.githubusercontent.com/getlantern/lantern-binaries/master/lantern-installer-beta.dmg](https://raw.githubusercontent.com/getlantern/lantern-binaries/master/lantern-installer-beta.dmg) Ubuntu 14.04 32 bit:[https://raw.githubusercontent.com/getlantern/lantern-binaries/master/lantern-installer-beta-32-bit.deb](https://raw.githubusercontent.com/getlantern/lantern-binaries/master/lantern-installer-beta-32-bit.deb) Ubuntu 14.04 64 bit: [https://raw.githubusercontent.com/getlantern/lantern-binaries/master/lantern-installer-beta-64-bit.deb](https://raw.githubusercontent.com/getlantern/lantern-binaries/master/lantern-installer-beta-64-bit.deb) 项目github地址:[https://github.com/getlantern/lantern](https://github.com/getlantern/lantern)
作者:微信小助手
<section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="padding-top: 10px;padding-right: 10px;padding-left: 10px;box-sizing: border-box;background-color: rgb(239, 239, 239);"> <span style="display: inline-block;width: 5%;line-height: 0.8;font-weight: bolder;font-size: 48px;box-sizing: border-box;"> <section style="box-sizing: border-box;"> “ </section></span> <section style="display: inline-block;vertical-align: top;float: right;width: 90%;line-height: 1.5;font-size: 15px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><span style="letter-spacing: 1px;">虽然在大家眼中,今天的互联网公司普遍以高科技和现代化著称,<span style="line-height: 1.5;box-sizing: border-box;">但实际上,在这些光荣与辉煌的背后,仍然存在着这样或那样的迷信现象。</span></span></p> </section> <section style="clear: both;box-sizing: border-box;"></section> </section> </section> </section> </section> <p style="line-height: 1.75em;"><br></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="0.5753424657534246" data-s="300,640" src="/upload/6d20bdc5a2e8f87be70426a0ad81fe7b.png" data-type="png" data-w="803" style=""></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">它们有的是遗留下来的古老传统,有的则是互联网化后的特色迷信。今天,就让我们共同探寻这些现象。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" data-backw="558" data-backh="558" data-before-oversubscription-url="/upload/7fcedf58cf3e9ad41d018666265d2869.jpg" src="/upload/7fcedf58cf3e9ad41d018666265d2869.jpg" style="line-height: 28px;text-align: justify;white-space: normal;width: 677px;box-sizing: border-box !important;word-wrap: break-word !important;visibility: visible !important;"></p> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyright 2018 iPaiban All Rights Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_imib_006')"> <section> <section> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;text-align: center;box-sizing: border-box;"> <section style="display: inline-block;border-radius: 16px;padding: 5px;vertical-align: top;line-height: 1;box-sizing: border-box;background-color: rgb(89, 89, 89);"> <section style="width: 18px;height: 18px;border: 4px solid rgb(255, 255, 255);border-top-left-radius: 50%;border-top-right-radius: 50%;border-bottom-right-radius: 50%;border-bottom-left-radius: 50%;box-sizing: border-box;display: inline-block;vertical-align: top;background-color: rgb(90, 89, 89);"></section> <section style="display: inline-block;vertical-align: top;color: rgb(255, 255, 255);padding-left: 6px;padding-right: 8px;height: 18px;line-height: 18px;font-size: 20px;box-sizing: border-box;"> <p style="box-sizing: border-box;">互联网公司迷信大全</p> </section> </section> </section> </section> </section> </section> </section> </section> </section> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" src="/upload/72a4dfd3738293af6d96b8027a0aa0bd.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 677px !important;visibility: visible !important;"></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="border-bottom-width: 1px;border-bottom-style: solid;border-bottom-color: black;margin-top: 0.5em;margin-bottom: 0.5em;line-height: 1.2;box-sizing: border-box;"> <section style="display: inline-block;border-bottom-width: 6px;border-bottom-style: solid;border-color: rgb(89, 89, 89);margin-bottom: -1px;font-size: 20px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;">表层迷信</p> </section> </section> </section> </section> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyright 2018 iPaiban All Rights Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_qmi_022')"> <section> <section class=""> <section class=""> <p style="line-height: normal;"><br></p> </section> </section> </section> </section> </section> <section xmlns="http://www.w3.org/1999/xhtml"> <section> <section> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="width: 0.6em;display: inline-block;vertical-align: middle;box-sizing: border-box;"> <span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.2;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span> <strong><span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.6;margin-top: 2px;margin-bottom: 2px;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span><span style="width: 0.6em;height: 0.6em;display: block;opacity: 1;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span></strong> </section> <section style="display: inline-block;vertical-align: middle;font-size: 18px;padding-left: 5px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><strong>拜神</strong></p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> </section> </section> </section> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">在一些互联网公司,你依然能看到财神、关公等传统神像被供奉。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">他们公司的类型无非这几种:</span></p> <ul class=" list-paddingleft-2" style="list-style-type: disc;"> <li><p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">传统企业转型</span></strong></p></li> <li><p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">老板听信“互联网思维”而半路出家</span></strong></p></li> <li><p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">老板有潮汕血统</span></strong></p></li> </ul> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">正所谓“互联网外衣虽穿在身,我心仍是外贸心”。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" data-backw="558" data-backh="558" data-before-oversubscription-url="/upload/cde04b3cba301556ea4ce0f5c1c13394.jpg" src="/upload/cde04b3cba301556ea4ce0f5c1c13394.jpg" style="width: 677px;box-sizing: border-box !important;word-wrap: break-word !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">不管处于怎样的环境,这些神像都会搭配中式茶台和成套茶具出现。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" data-backw="558" data-backh="558" data-before-oversubscription-url="/upload/bc4c51abd0fa353fe12624eec319cc67.jpg" src="/upload/bc4c51abd0fa353fe12624eec319cc67.jpg" style="text-align: center;box-sizing: border-box !important;word-wrap: break-word !important;width: 558px !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">真正的原生互联网企业都崇拜新神。</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">与《美国众神》类似,在互联网企业的世界观里,旧神垂垂老矣,且所分管的领域早已不适应时代发展;只有新神才能保佑他们。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" data-backw="558" data-backh="558" data-before-oversubscription-url="/upload/5f9a8d8a46a4a2bb7b88a8fb648df667.jpg" src="/upload/5f9a8d8a46a4a2bb7b88a8fb648df667.jpg" style="text-align: center;box-sizing: border-box !important;word-wrap: break-word !important;width: 558px !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">新神大都不是虚无缥缈的人物,他们属于人间,有着足够的曝光率,且不少都是该领域最大平台的老大。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><img class="" data-backh="558" data-backw="558" data-before-oversubscription-url="https://mmbiz.qpic.cn/mmbiz_jpg/W9YcQcK245bzBuiaibNXicdKgMbJgTOcCvRQJpcm2VrSwCZORicDbLmfMbreurVpBIezgnjVCbfbcdOC9LbOTAe65g/0?wx_fmt=jpeg" data-copyright="0" data-cropselx1="0" data-cropselx2="558" data-cropsely1="0" data-cropsely2="558" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="600" src="/upload/c7cce64b4814a49bce1f4236561f1fb2.jpg" style="width: 677px;box-sizing: border-box !important;word-wrap: break-word !important;visibility: visible !important;"></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 14px;"><em><span style="line-height: 25.6px;color: rgb(89, 89, 89);letter-spacing: 1px;">马云、刘强东——电商之神</span></em></span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-backh="558" data-backw="558" data-before-oversubscription-url="https://mmbiz.qpic.cn/mmbiz_jpg/W9YcQcK245bzBuiaibNXicdKgMbJgTOcCvRgLyVrghzmPYuib4yox8ZvcTOhhadwgDYZYqQVibxCViavQibMRAgdpROqA/0?wx_fmt=jpeg" data-copyright="0" data-cropselx1="0" data-cropselx2="558" data-cropsely1="0" data-cropsely2="558" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="600" src="/upload/4dd72712c9f0e9608fe47ee8b811011c.jpg" style="width: 677px;box-sizing: border-box !important;word-wrap: break-word !important;visibility: visible !important;"></p> <p style="text-align: center;margin-bottom: 5px;"><span style="font-size: 14px;"><em><span style="color: rgb(89, 89, 89);letter-spacing: 1px;line-height: 28px;text-align: justify;">张小龙——公众号之神</span></em></span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-backh="558" data-backw="558" data-before-oversubscription-url="https://mmbiz.qpic.cn/mmbiz_jpg/W9YcQcK245bzBuiaibNXicdKgMbJgTOcCvRbKbrVOUjgLoZRRyyScMasskuoclrjqWlXm8FFPqFcpiaE5ydZ2HxC9Q/0?wx_fmt=jpeg" data-copyright="0" data-cropselx1="0" data-cropselx2="558" data-cropsely1="0" data-cropsely2="558" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="600" src="/upload/96b42cd62f2a2d901fe5a32a7abbe662.jpg" style="width: 677px;box-sizing: border-box !important;word-wrap: break-word !important;visibility: visible !important;"></p> <p style="text-align: center;"><span style="font-size: 14px;"><em><span style="color: rgb(89, 89, 89);letter-spacing: 1px;line-height: 28px;text-align: justify;">来去之间——微博之神</span></em></span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">互联网造神的速度就和它的风口变化一样快。</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">一篇微信推送创造出的活体锦鲤可能几天就被遗忘了。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-backh="558" data-backw="558" data-before-oversubscription-url="https://mmbiz.qpic.cn/mmbiz_jpg/W9YcQcK245bzBuiaibNXicdKgMbJgTOcCvRBWzCshNrE5dnmhYajwrMGHjxgeicBz4L4L710DI21glYGibznqRzbKVQ/0?wx_fmt=jpeg" data-copyright="0" data-cropselx1="0" data-cropselx2="558" data-cropsely1="0" data-cropsely2="558" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="600" src="/upload/79994edb3b7a2d47668125b65fef329b.jpg" style="width: 677px;box-sizing: border-box !important;word-wrap: break-word !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><strong><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">此外,还有一类因“谐音”上位的神:</span></strong><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">雍正就因为专治八阿哥(Bug)而被挂在程序员办公区。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" data-backw="558" data-backh="558" data-before-oversubscription-url="/upload/1493bb89b1dfeaef7a518eb47e2d1e1c.jpg" src="/upload/1493bb89b1dfeaef7a518eb47e2d1e1c.jpg" style="width: 677px;box-sizing: border-box !important;word-wrap: break-word !important;visibility: visible !important;"></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="width: 0.6em;display: inline-block;vertical-align: middle;box-sizing: border-box;"> <span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.2;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span> <span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.6;margin-top: 2px;margin-bottom: 2px;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span> <strong><span style="width: 0.6em;height: 0.6em;display: block;opacity: 1;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span></strong> </section> <section style="display: inline-block;vertical-align: middle;font-size: 18px;padding-left: 5px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><strong>祭祀</strong></p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">从“暴风影音”开始,互联网公司在一段时间内更新 App 时都喜欢“杀一个程序员祭天”。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">虽然我们不知道具体是怎样执行的,但这项仪式似乎随着版本更新越来越频繁而废止;也有人说是因为“Bug 之神”的愤怒并没有因为类似祭祀行为而消减。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" data-backw="558" data-backh="558" data-before-oversubscription-url="/upload/78a4bc249d0559ab408390f42ece4f03.jpg" src="/upload/78a4bc249d0559ab408390f42ece4f03.jpg" style="width: 677px;box-sizing: border-box !important;word-wrap: break-word !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">而前两年 B 站乔迁新办公楼,弄了个大的道场,祭祀仪式也搞得有模有样,法台、祭品、鲜花、黄符、道士一应俱全。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" data-backw="558" data-backh="558" data-before-oversubscription-url="/upload/5b23b25a2f650ac45d68f17325ee17.jpg" src="/upload/5b23b25a2f650ac45d68f17325ee17.jpg" style="width: 677px;box-sizing: border-box !important;word-wrap: break-word !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">在这方面,二次元新人类还得依靠传统三次元的力量,毕竟最后奔的是资本市场而不是玉子市场。</span></p> <p style="line-height: normal;"><br></p> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyleft 2018 iPaiban All lefts Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_gtitle_011')"> <section> <section> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="width: 0.6em;display: inline-block;vertical-align: middle;box-sizing: border-box;"> <span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.2;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span> <strong><span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.6;margin-top: 2px;margin-bottom: 2px;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span><span style="width: 0.6em;height: 0.6em;display: block;opacity: 1;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span></strong> </section> <section style="display: inline-block;vertical-align: middle;font-size: 18px;padding-left: 5px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><strong>开光</strong></p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> </section> </section> </section> </section> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">技术部门在这个领域当之无愧走在了前列。</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">作为许多互联网公司的命脉,服务器的稳定是重中之重,因此服务器开光也成了重要一环。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">为了达到预期效果,他们有的直接抬着服务器去寺庙进行开光,并由程序员全程护送。</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">原产地直供,更安全。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-backh="558" data-backw="558" data-before-oversubscription-url="https://mmbiz.qpic.cn/mmbiz_jpg/W9YcQcK245bzBuiaibNXicdKgMbJgTOcCvRuWmvutsqdbmE080eF6QlyWxPNt7ZgObNPkybX8UvUuV7Ny6Gglp0eA/0?wx_fmt=jpeg" data-copyright="0" data-cropselx1="0" data-cropselx2="558" data-cropsely1="0" data-cropsely2="558" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="800" src="/upload/9e546dd18905b08ec0a1ac6ef989ef79.jpg" style="width: 677px;box-sizing: border-box !important;word-wrap: break-word !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">如果没办法送庙里,就要用最贴近本职工作的方式来完成这项仪式。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">比如用“行话”:</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" src="/upload/eabc4f2c2253bdd78869cb9b8090d835.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 677px !important;visibility: visible !important;"></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" src="/upload/c68e39be34bc4aba0eb9383478f7aab4.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 677px !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">再比如用“深层行话+象形图案”:</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" src="/upload/c2c063ecadb55195291450ea6d7b2fb1.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 677px !important;visibility: visible !important;"></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="border-bottom-width: 1px;border-bottom-style: solid;border-bottom-color: black;margin-top: 0.5em;margin-bottom: 0.5em;line-height: 1.2;box-sizing: border-box;"> <section style="display: inline-block;border-bottom-width: 6px;border-bottom-style: solid;border-color: rgb(89, 89, 89);margin-bottom: -1px;font-size: 20px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;">中层迷信</p> </section> </section> </section> </section> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyright 2018 iPaiban All Rights Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_qmi_022')"> <section> <section class=""> <section class=""> <p style="line-height: normal;"><br></p> </section> </section> </section> </section> </section> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyleft 2018 iPaiban All lefts Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_gtitle_011')"> <section> <section> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="width: 0.6em;display: inline-block;vertical-align: middle;box-sizing: border-box;"> <span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.2;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span> <strong><span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.6;margin-top: 2px;margin-bottom: 2px;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span><span style="width: 0.6em;height: 0.6em;display: block;opacity: 1;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span></strong> </section> <section style="display: inline-block;vertical-align: middle;font-size: 18px;padding-left: 5px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><strong>数字迷信</strong></p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> </section> </section> </section> </section> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">产品一定要在某个精确的时间点上线或改版,仿佛错过了这个时间整个项目就会失败。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="0.74" data-s="300,640" data-type="jpeg" data-w="1000" src="/upload/3365cf71731039101823c848fe219f52.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 677px !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">然而老板的幸运数字一般情况下不太幸运,拿去买彩票也中不了奖。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" src="/upload/d7f4c3d080ffecfe3025632388761057.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 677px !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;letter-spacing: 1px;color: rgb(71, 193, 168);">数字域名里要带“6”和“8”:</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" src="/upload/47c288790c8b7b3d023742d2f96b4f28.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 677px !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">公司和产品起名字之前要测一下分数,数值低于 80 直接被否。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" src="/upload/3e1b740662b147045efdef1a57fdc8c9.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 677px !important;visibility: visible !important;"></p> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyleft 2018 iPaiban All lefts Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_gtitle_011')"> <section> <section> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="width: 0.6em;display: inline-block;vertical-align: middle;box-sizing: border-box;"> <span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.2;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span> <strong><span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.6;margin-top: 2px;margin-bottom: 2px;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span><span style="width: 0.6em;height: 0.6em;display: block;opacity: 1;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span></strong> </section> <section style="display: inline-block;vertical-align: middle;font-size: 18px;padding-left: 5px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><strong>星座迷信</strong></p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> </section> </section> </section> </section> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">因为摩羯座就是为工作而生的星座,很多公司招人时大量启用摩羯座,导致在 12 月底到 1 月中要频繁举行员工生日下午茶(当然也可以一次多人过生日节省成本)。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">当然,时常也会有“你是个假摩羯座吧”的感慨。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="600" src="/upload/5a8b0bbdd1f54cee0c9940c4bde90560.jpg" style="text-align: center;box-sizing: border-box !important;word-wrap: break-word !important;width: 600px !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">同样,会觉得某些星座天生适合做某项工作,比如射手座阳光开朗做商务,处女座完美主义做设计......天蝎和巨蟹不适合工作。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" src="/upload/e47be83c12e35845cbe584f45e4187a0.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 677px !important;visibility: visible !important;"></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img data-copyright="0" data-s="300,640" data-type="jpeg" class="" data-ratio="1" data-w="600" src="/upload/3119988b55f2a20cee1d79aeee31cdaa.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 600px !important;visibility: visible !important;"></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-cropselx1="0" data-cropselx2="558" data-cropsely1="0" data-cropsely2="558" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="600" src="/upload/cbcfcf981630840bf4e579ca0b4586d2.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 558px !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">总之,星座不会错,错的是人。</span></p> <p style="line-height: normal;"><br></p> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyleft 2018 iPaiban All lefts Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_gtitle_011')"> <section> <section> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="width: 0.6em;display: inline-block;vertical-align: middle;box-sizing: border-box;"> <span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.2;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span> <strong><span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.6;margin-top: 2px;margin-bottom: 2px;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span><span style="width: 0.6em;height: 0.6em;display: block;opacity: 1;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span></strong> </section> <section style="display: inline-block;vertical-align: middle;font-size: 18px;padding-left: 5px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><strong>面相迷信</strong></p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> </section> </section> </section> </section> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">看简历时以照片先入为主,面试时虽然问了很多问题但最后还是以面相来判断一个人是否合适。“有些人说不清哪里不好,但就是面相合适不了。”</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="600" src="/upload/97f36c2515b4e7a12de86807826fb34f.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 600px !important;visibility: visible !important;"></p> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyleft 2018 iPaiban All lefts Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_gtitle_011')"> <section> <section> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="width: 0.6em;display: inline-block;vertical-align: middle;box-sizing: border-box;"> <span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.2;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span> <strong><span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.6;margin-top: 2px;margin-bottom: 2px;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span><span style="width: 0.6em;height: 0.6em;display: block;opacity: 1;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span></strong> </section> <section style="display: inline-block;vertical-align: middle;font-size: 18px;padding-left: 5px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><strong>社交迷信</strong></p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> </section> </section> </section> </section> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">每个 App 都有一颗不死的做社交的心,甭管是内容产品还是工具产品;</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">哪怕前方是万丈深渊,哪怕结果是万劫不复。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="600" src="/upload/ee01742b0e101dee6051ef9ed4932a11.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 600px !important;visibility: visible !important;"></p> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyleft 2018 iPaiban All lefts Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_gtitle_011')"> <section> <section> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="width: 0.6em;display: inline-block;vertical-align: middle;box-sizing: border-box;"> <span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.2;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span> <strong><span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.6;margin-top: 2px;margin-bottom: 2px;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span><span style="width: 0.6em;height: 0.6em;display: block;opacity: 1;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span></strong> </section> <section style="display: inline-block;vertical-align: middle;font-size: 18px;padding-left: 5px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><strong>穿衣迷信</strong></p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> </section> </section> </section> </section> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">只穿某个颜色的衣服;只有某种款式的衣服;</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">在关键时刻和重要场合只穿那一套可以给自己带来幸运的衣服。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1.5" data-s="300,640" data-type="jpeg" data-w="600" data-backw="558" data-backh="837" data-before-oversubscription-url="/upload/28a7f61ad6cd6095f2174c27266385e6.jpg" src="/upload/28a7f61ad6cd6095f2174c27266385e6.jpg" style="width: 677px;box-sizing: border-box !important;word-wrap: break-word !important;visibility: visible !important;"></p> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyright 2018 iPaiban All Rights Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_qmi_022')"> <section> <section class=""> <section class=""> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="border-bottom-width: 1px;border-bottom-style: solid;border-bottom-color: black;margin-top: 0.5em;margin-bottom: 0.5em;line-height: 1.2;box-sizing: border-box;"> <section style="display: inline-block;border-bottom-width: 6px;border-bottom-style: solid;border-color: rgb(89, 89, 89);margin-bottom: -1px;font-size: 20px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;">深层迷信</p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> </section> </section> </section> </section> </section> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyleft 2018 iPaiban All lefts Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_gtitle_011')"> <section> <section> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="width: 0.6em;display: inline-block;vertical-align: middle;box-sizing: border-box;"> <span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.2;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span> <strong><span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.6;margin-top: 2px;margin-bottom: 2px;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span><span style="width: 0.6em;height: 0.6em;display: block;opacity: 1;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span></strong> </section> <section style="display: inline-block;vertical-align: middle;font-size: 18px;padding-left: 5px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><strong>风口</strong></p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> </section> </section> </section> </section> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">深深迷信“风口上的猪”言论,一旦有新的风口出现立刻涉猎或转型,美其名曰“战略调整”,生怕赶不上趟。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">如果失败会将原因归结为“还不够敏锐”,并且暗自下决心新的风口出现前提高“商业嗅觉”。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="600" src="/upload/a0a6646565d272d7f76a31b2c4982ab.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 600px !important;visibility: visible !important;"></p> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyleft 2018 iPaiban All lefts Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_gtitle_011')"> <section> <section> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="width: 0.6em;display: inline-block;vertical-align: middle;box-sizing: border-box;"> <span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.2;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span> <strong><span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.6;margin-top: 2px;margin-bottom: 2px;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span><span style="width: 0.6em;height: 0.6em;display: block;opacity: 1;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span></strong> </section> <section style="display: inline-block;vertical-align: middle;font-size: 18px;padding-left: 5px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><strong>风水</strong></p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> </section> </section> </section> </section> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">阿里巴巴西溪园区只允许建七层,因为“七上八下”,再往上就不吉利了。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">腾讯北京总部做成了个大船的形状,因为在这个“中国互联网的十字路口”分别有百度、新浪和网易,而船可以撒网(易),用来摆渡(百度),还不怕(新)浪。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">都说互联网行业是属火的,所以千万不能沾到水。</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">望京和酒仙桥因地处北京东北为艮属土,且有坝河流过,水气充足,兼备“火生土和水克火”, 让无数互联网公司折戟沉沙。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="600" src="/upload/d1cd4b3d32ac7b09a237931b26fceb40.jpg" style="text-align: center;box-sizing: border-box !important;word-wrap: break-word !important;width: 600px !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">因此,望京和酒仙桥也分别被冠以“互联网滑铁卢“和“互联网百慕大”。</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">而整个上海干脆因为长江环绕,且作为长江入海口不能聚流,被认定压根就不适合互联网公司生存。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">反观中关村理想国际大厦,因为曾经历过百度、新浪、优酷土豆、蔚来等众多互联网企业的辉煌时刻被普遍认为是一块“风水宝地”。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">不过前阵子,OFO 团队因为交不起租金刚刚从这座大厦搬离了。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" src="/upload/d28221a19342f731fdfda98b48cfe7b9.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 677px !important;visibility: visible !important;"></p> <section xmlns="http://www.w3.org/1999/xhtml"> <section label="Copyleft 2018 iPaiban All lefts Reserved (本样式已做版权保护,未经正式授权不允许任何第三方编辑器、企业、个人使用,违者必纠)" donone="shifuMouseDownPayStyle('shifu_gtitle_011')"> <section> <section> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 10px;margin-bottom: 10px;box-sizing: border-box;"> <section style="width: 0.6em;display: inline-block;vertical-align: middle;box-sizing: border-box;"> <span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.2;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span> <strong><span style="width: 0.6em;height: 0.6em;display: block;opacity: 0.6;margin-top: 2px;margin-bottom: 2px;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span><span style="width: 0.6em;height: 0.6em;display: block;opacity: 1;box-sizing: border-box;background-color: rgb(89, 89, 89);"></span></strong> </section> <section style="display: inline-block;vertical-align: middle;font-size: 18px;padding-left: 5px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;"><strong>朝圣</strong></p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> </section> </section> </section> </section> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">不少中国互联网人的心中都有一个神圣的地方——硅谷;</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">那里就是互联网人的麦加。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">谷歌总部、苹果总部、脸书........顺道还能去趟斯坦福大学;</span><span style="color: rgb(89, 89, 89);font-size: 15px;letter-spacing: 1px;line-height: 1.75em;">总之,该看的一个都不能漏。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">一路朝圣还要不停发朋友圈,三步拍一张图,五步感慨一段文字,好比西藏跪拜式朝圣。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" src="/upload/bd45a0fecedd0cc8b75f2ea77f3824a9.jpg" style="text-align: center;box-sizing: border-box !important;word-wrap: break-word !important;width: 677px !important;visibility: visible !important;"></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">乔布斯则是圣人中的圣人。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">还有上市了的互联网大佬把房子买在了乔布斯故居旁边,呼吸着他曾经呼吸过的空气,在每一寸土地感受他的存在,幻想着说不定哪天他老人家还能显灵,和自己聊一下他对于 iPhone XS MAX 的看法。</span></p> <p style="text-align: center;margin-left: 8px;margin-right: 8px;line-height: 1.75em;margin-bottom: 5px;"><img class="" data-copyright="0" data-ratio="1" data-s="300,640" data-type="jpeg" data-w="1000" src="/upload/d1f3dca1eb9740d4510de3e90290f788.jpg" style="box-sizing: border-box !important;word-wrap: break-word !important;width: 677px !important;visibility: visible !important;"></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="border-bottom-width: 1px;border-bottom-style: solid;border-bottom-color: black;margin-top: 0.5em;margin-bottom: 0.5em;line-height: 1.2;box-sizing: border-box;"> <section style="display: inline-block;border-bottom-width: 6px;border-bottom-style: solid;border-color: rgb(89, 89, 89);margin-bottom: -1px;font-size: 20px;color: rgb(89, 89, 89);box-sizing: border-box;"> <p style="box-sizing: border-box;">结语</p> </section> </section> </section> </section> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">商业世界风云莫测、前途迷茫,当创业者安全感低时,会寄希望于超自然寻求心灵慰藉。</span></p> <p style="line-height: normal;"><br></p> <p style="text-align: justify;margin-left: 8px;margin-right: 8px;line-height: 1.75em;"><span style="font-size: 15px;color: rgb(89, 89, 89);letter-spacing: 1px;">但这个世界只有因果,没有迷信。求神不如求己。希望你能克服一切困难历经一切风霜后最终拥抱成功。</span></p> <p style="line-height: normal;"><br></p> <p style="white-space: normal;text-align: justify;line-height: 1.75em;"><span style="color: rgb(89, 89, 89);letter-spacing: 1px;"><em><span style="font-size: 14px;">作者:<span style="line-height: 25.6px;">crossoverJie</span></span></em></span></p> <p style="white-space: normal;text-align: justify;line-height: 1.75em;"><span style="color: rgb(89, 89, 89);letter-spacing: 1px;"><em><span style="font-size: 14px;">编辑:陶家龙、孙淑娟</span></em></span><br></p> <p style="text-align: justify;line-height: 1.75em;margin-bottom: 5px;"><span style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;"><em>出处:本文经授权转载自微信公众号“吓脑湿”(imyourgrandpa)。</em></span></p> <section class="" style="line-height: 25.6px;white-space: normal;"> <section class="" data-source="bj.96weixin.com" style="max-width: 100%;color: rgb(51, 51, 51);font-family: -apple-system-font, BlinkMacSystemFont, 'Helvetica Neue', 'PingFang SC', 'Hiragino Sans GB', 'Microsoft YaHei UI', 'Microsoft YaHei', Arial, sans-serif;font-size: 17px;letter-spacing: 0.544px;text-align: justify;line-height: 25.6px;box-sizing: border-box !important;word-wrap: break-word !important;background-color: rgb(255, 255, 255);"> <section style="margin-top: 5px;margin-bottom: 5px;max-width: 100%;box-sizing: border-box !important;word-wrap: break-word !important;"> <section class="" style="margin: 10px auto;max-width: 100%;border-style: solid;-webkit-border-image: url("https://mmbiz.qpic.cn/mmbiz/z9433rAGTDfghx2FYIqPBl4tcLzFZiaXJfibf6EgwbxL5HiayCvl5qHPl7ylDwgZIErc203rh9bHJ6jcMmMPCfdUQ/640?wx_fmt=png") 145 60 40 400 fill;border-width: 40px 15px 15px 80px;box-sizing: border-box !important;word-wrap: break-word !important;"> <section style="max-width: 100%;box-sizing: border-box !important;word-wrap: break-word !important;"> <p style="max-width: 100%;min-height: 1em;text-align: center;box-sizing: border-box !important;word-wrap: break-word !important;"><span style="max-width: 100%;box-sizing: border-box !important;word-wrap: break-word !important;"><strong style="max-width: 100%;box-sizing: border-box !important;word-wrap: break-word !important;"><span style="max-width: 100%;color: rgb(255, 104, 39);box-sizing: border-box !important;word-wrap: break-word !important;">留 言 有 礼 活 动</span></strong></span></p> <p style="max-width: 100%;min-height: 1em;box-sizing: border-box !important;word-wrap: break-word !important;"><span style="max-width: 100%;font-size: 14px;box-sizing: border-box !important;word-wrap: break-word !important;"><strong style="max-width: 100%;box-sizing: border-box !important;word-wrap: break-word !important;"><span style="max-width: 100%;color: rgb(89, 89, 89);line-height: 25.6px;box-sizing: border-box !important;word-wrap: break-word !important;">作为 IT 技术人,你焦虑过吗?你会如何克服现有的焦虑?</span></strong><span style="max-width: 100%;color: rgb(89, 89, 89);line-height: 25.6px;">小编将精选出最有价值的三条评论,分别获得 <strong style="max-width: 100%;box-sizing: border-box !important;word-wrap: break-word !important;">50、30、20 元 的 红 包 奖 励</strong>,活动截止时间 12 月 21 号 12 时整。</span></span></p> </section> </section> </section> </section> </section> <p style="text-align: center;"><img class="" data-copyright="0" data-ratio="0.3939393939393939" src="/upload/70dc65115d35e7d4c78cd84ded7bacac.gif" data-type="gif" data-w="660" style=""></p> <p style="text-align: center;"> <mp-miniprogram class="miniprogram_element" data-miniprogram-appid="wxc58e6e4759a08388" data-miniprogram-path="pages/index" data-miniprogram-nickname="51CTO播客" data-miniprogram-avatar="http://mmbiz.qpic.cn/mmbiz_png/M3DmwKCWDnP2VudjoA84HWJSg9Ige9Gfy7AKjdzNgmUkbYqIEIagHNoAGmSBibBxEMRNEjOJJArsoFchiakzmtxg/640?wx_fmt=png&wxfrom=200" data-miniprogram-title="51CTO播客,随时随地,碎片化学习" data-miniprogram-imageurl="http://mmbiz.qpic.cn/mmbiz_jpg/MOwlO0INfQrQfB8hW7DLAkiav1icdWOplv0AXq9oCOa1y0AOJFcyQdHulKDPzoezj8ap3fKKWPt6sHYdYQQqnZ0A/0?wx_fmt=jpeg"></mp-miniprogram></p> <section style="box-sizing: border-box;"> <section class="V5" style="box-sizing: border-box;" powered-by="xiumi.us"> <section style="margin-top: 0.5em;margin-bottom: 0.5em;box-sizing: border-box;"> <section style="font-size: 15px;border-style: solid;border-width: 0px 0px 1px;color: rgb(89, 89, 89);border-bottom-color: rgba(215, 215, 215, 0.960784);box-sizing: border-box;"> <p style="box-sizing: border-box;"><span style="letter-spacing: 1px;"><strong>精彩文章推荐:</strong></span></p> </section> </section> </section> </section> <p style="text-align: justify;line-height: 2em;"><a href="http://mp.weixin.qq.com/s?__biz=MjM5ODI5Njc2MA==&mid=2655821269&idx=1&sn=4de76f19c2e845597a7d26cbd9c53c1d&chksm=bd74d0028a0359141530f888b9b82dc8fc72285ae9dc20598183f99f8404cddc3c0877519841&scene=21#wechat_redirect" target="_blank" style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;text-decoration: none;"><span style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;">没想到《天龙八部》这段,只有搞IT的才懂</span></a><br></p> <p style="text-align: justify;line-height: 2em;"><a href="http://mp.weixin.qq.com/s?__biz=MjM5ODI5Njc2MA==&mid=2655821398&idx=2&sn=0ef1df344348d6485efe6ef674240143&chksm=bd74d3818a035a976c0f6910c260718e0a0345783898106cf986b73969bfbfb49de18cdb7ae5&scene=21#wechat_redirect" target="_blank" style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;text-decoration: none;"><span style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;">技术变化那么快,程序员如何做到不被淘汰?</span></a><br></p> <p style="text-align: justify;line-height: 2em;"><a href="http://mp.weixin.qq.com/s?__biz=MjM5ODI5Njc2MA==&mid=2655821313&idx=1&sn=123b25ca90fe06aa404339f2dd89855e&chksm=bd74d3d68a035ac095da842e32865d904e2f7ec90ad93180eda421233608191280e45104eb33&scene=21#wechat_redirect" target="_blank" style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;text-decoration: none;"><span style="font-size: 14px;color: rgb(89, 89, 89);letter-spacing: 1px;">还不理解“分布式事务”?这篇给你讲清楚!</span></a><br></p>
作者:じ☆ve宝贝
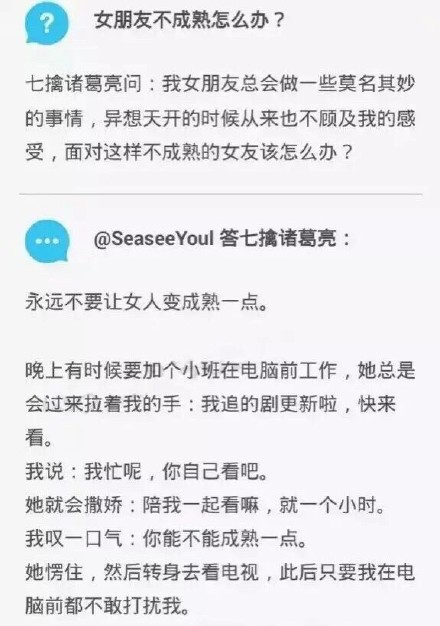 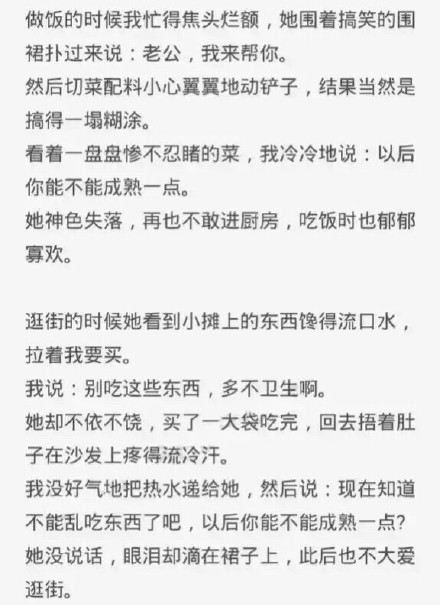 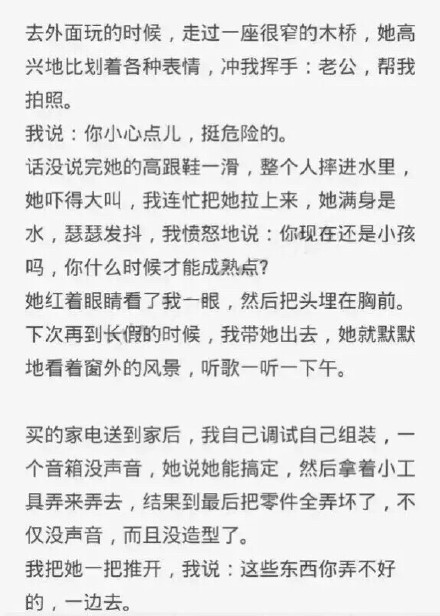 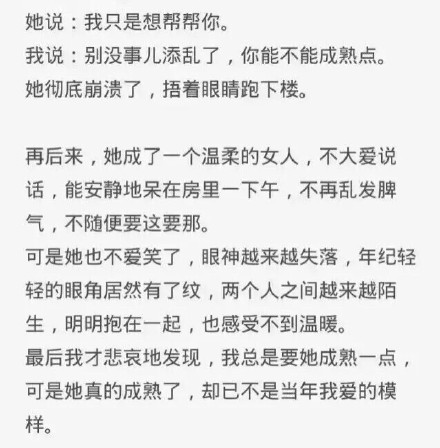 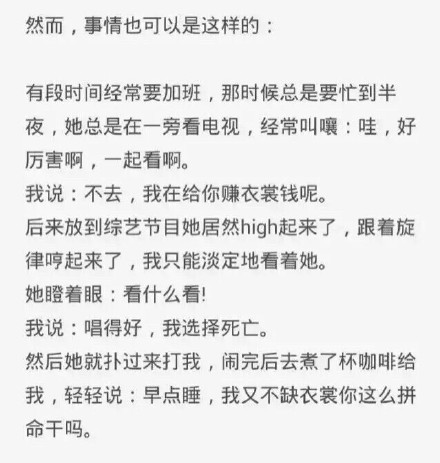 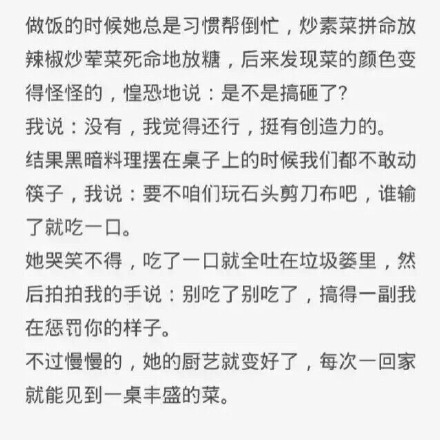  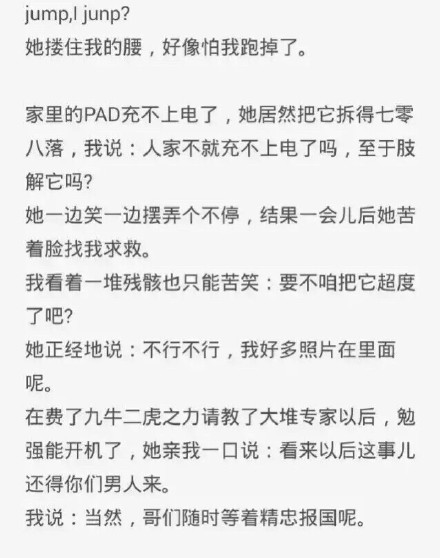 